Create Bouncing Ball Game in Python With Source Code
Last updated June 2, 2023 by Jarvis Silva
Looking for a way to create a bouncing ball game in python, then you are at the right place today. In this python tutorial we will see how to create a simple bouncing ball game using our python skills.
In this game there are several ball-like shaped things at the top, the ball hits them and comes at the ground and you have to move the ground plane where the ball will land, if you miss the ball then game over.
To create this bouncing ball game we will use the tkinter library, It is a GUI library which can be used for developing graphics in python.
Bouncing Ball Game Code In Python
from tkinter import *
import time
import random
root = Tk()
root.title("Bounce Ball")
root.geometry("500x570")
root.resizable(0, 0)
root.wm_attributes("-topmost", 1)
canvas = Canvas(root, width=500, height=500, bd=0, highlightthickness=0, highlightbackground="Red", bg="Black")
canvas.pack(padx=10, pady=10)
score = Label(height=50, width=80, text="Score: 00", font="Consolas 14 bold")
score.pack(side="left")
root.update()
class Ball:
def __init__(self, canvas, color, paddle, bricks, score):
self.bricks = bricks
self.canvas = canvas
self.paddle = paddle
self.score = score
self.bottom_hit = False
self.hit = 0
self.id = canvas.create_oval(10, 10, 25, 25, fill=color, width=1)
self.canvas.move(self.id, 230, 461)
start = [4, 3.8, 3.6, 3.4, 3.2, 3, 2.8, 2.6]
random.shuffle(start)
#print(start)
self.x = start[0]
self.y = -start[0]
self.canvas.move(self.id, self.x, self.y)
self.canvas_height = canvas.winfo_height()
self.canvas_width = canvas.winfo_width()
def brick_hit(self, pos):
for brick_line in self.bricks:
for brick in brick_line:
brick_pos = self.canvas.coords(brick.id)
#print(brick_pos)
try:
if pos[2] >= brick_pos[0] and pos[0] <= brick_pos[2]:
if pos[3] >= brick_pos[1] and pos[1] <= brick_pos[3]:
canvas.bell()
self.hit += 1
self.score.configure(text="Score: " + str(self.hit))
self.canvas.delete(brick.id)
return True
except:
continue
return False
def paddle_hit(self, pos):
paddle_pos = self.canvas.coords(self.paddle.id)
if pos[2] >= paddle_pos[0] and pos[0] <= paddle_pos[2]:
if pos[3] >= paddle_pos[1] and pos[1] <= paddle_pos[3]:
#print("paddle hit")
return True
return False
def draw(self):
self.canvas.move(self.id, self.x, self.y)
pos = self.canvas.coords(self.id)
#print(pos)
start = [4, 3.8, 3.6, 3.4, 3.2, 3, 2.8, 2.6]
random.shuffle(start)
if self.brick_hit(pos):
self.y = start[0]
if pos[1] <= 0:
self.y = start[0]
if pos[3] >= self.canvas_height:
self.bottom_hit = True
if pos[0] <= 0:
self.x = start[0]
if pos[2] >= self.canvas_width:
self.x = -start[0]
if self.paddle_hit(pos):
self.y = -start[0]
class Paddle:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_rectangle(0, 0, 100, 10, fill=color)
self.canvas.move(self.id, 200, 485)
self.x = 0
self.pausec=0
self.canvas_width = canvas.winfo_width()
self.canvas.bind_all("", self.turn_left)
self.canvas.bind_all("", self.turn_right)
self.canvas.bind_all("", self.pauser)
def draw(self):
pos = self.canvas.coords(self.id)
#print(pos)
if pos[0] + self.x <= 0:
self.x = 0
if pos[2] + self.x >= self.canvas_width:
self.x = 0
self.canvas.move(self.id, self.x, 0)
def turn_left(self, event):
self.x = -3.5
def turn_right(self, event):
self.x = 3.5
def pauser(self,event):
self.pausec+=1
if self.pausec==2:
self.pausec=0
class Bricks:
def __init__(self, canvas, color):
self.canvas = canvas
self.id = canvas.create_oval(5, 5, 25, 25, fill=color, width=2)
playing = False
def start_game(event):
global playing
if playing is False:
playing = True
score.configure(text="Score: 00")
canvas.delete("all")
BALL_COLOR = ["red", "yellow", "white"]
BRICK_COLOR = ["PeachPuff3", "dark slate gray", "rosy brown", "light goldenrod yellow", "turquoise3", "salmon",
"light steel blue", "dark khaki", "pale violet red", "orchid", "tan", "MistyRose2",
"DodgerBlue4", "wheat2", "RosyBrown2", "bisque3", "DarkSeaGreen1"]
random.shuffle(BALL_COLOR)
paddle = Paddle(canvas, "blue")
bricks = []
for i in range(0, 5):
b = []
for j in range(0, 19):
random.shuffle(BRICK_COLOR)
tmp = Bricks(canvas, BRICK_COLOR[0])
b.append(tmp)
bricks.append(b)
for i in range(0, 5):
for j in range(0, 19):
canvas.move(bricks[i][j].id, 25 * j, 25 * i)
ball = Ball(canvas, BALL_COLOR[0], paddle, bricks, score)
root.update_idletasks()
root.update()
time.sleep(1)
while 1:
if paddle.pausec !=1:
try:
canvas.delete(m)
del m
except:
pass
if not ball.bottom_hit:
ball.draw()
paddle.draw()
root.update_idletasks()
root.update()
time.sleep(0.01)
if ball.hit==95:
canvas.create_text(250, 250, text="YOU WON !!", fill="yellow", font="Consolas 24 ")
root.update_idletasks()
root.update()
playing = False
break
else:
canvas.create_text(250, 250, text="GAME OVER!!", fill="red", font="Consolas 24 ")
root.update_idletasks()
root.update()
playing = False
break
else:
try:
if m==None:pass
except:
m=canvas.create_text(250, 250, text="PAUSE!!", fill="green", font="Consolas 24 ")
root.update_idletasks()
root.update()
root.bind_all("", start_game)
canvas.create_text(250, 250, text="Press Enter to start Game!!", fill="red", font="Consolas 18")
j=canvas.find_all()
root.mainloop()
Above is the python code for the bouncing ball game, if you look at the code you will see a lot of tkinter functions so let’s how do they work:
- The code imports the necessary modules:
Tkinter
,time
, andrandom
. - The root window is created using
Tk()
and various attributes are set, such as the title, size, and visibility. - A canvas widget is created to serve as the game board.
- A label widget is created to display the score.
- The
Ball
class is defined, which represents the bouncing ball in the game. It has methods to handle collision detection with bricks and the paddle, and to update its position. - The
Paddle
class is defined, representing the player-controlled paddle. It has methods to handle movement and pause functionality. - The
Bricks
class is defined to create bricks on the game board. - The
start_game
function is defined, which initializes the game when the Enter key is pressed. It creates instances of the paddle, bricks, and ball, and manages the game loop. - Event bindings are set up to start the game and control the paddle movement.
- The main event loop is started using
root.mainloop()
, which keeps the game running.
Before running you need to install tkinter as we have used it to install use the below command
pip install tkinter
When you run this program It will open a new window and it will ask you to press enter key to start the game, below is image output of the game.
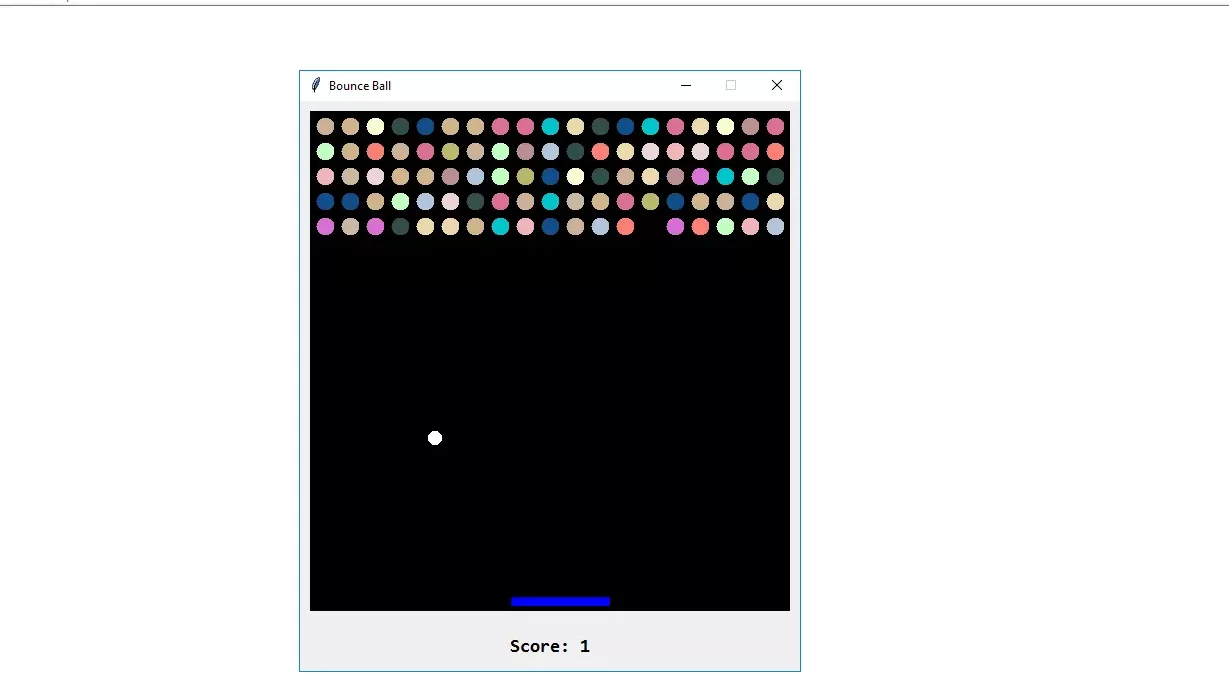
You can play this game with your side arrow keys left and right to move the plane you need to bounce the ball on the plane and if you miss it game over.
If you ran it successfully you will see we created the game successfully I hope you found this tutorial helpful and useful, Do share this with your friends who might be interested in this game.
Here are some more python game tutorials you will find interesting:
- Create pacman game in python with code.
- Create minecraft game clone in python.
- Create Fruit ninja game in python with code.
I hope you found what you were looking for from this tutorial and if you want more tutorials like this then do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂