Draw Square In Python Without Turtle
Last updated June 21, 2023 by Jarvis Silva
Today in this article we will see how to draw a square in python without turtle library which is a GUI library in python which can be used to draw things so we will not use it for drawing a square.
I will show you two methods to draw square one by using the pygame library which is a game development library in python and another using for loop.
Python Code For Drawing Square Using Pygame
import pygame
# Initialize Pygame
pygame.init()
# Setting window and color
size= 400,400
white_color = 255, 255, 255
screen = pygame.display.set_mode(size)
screen.fill(white_color)
# Set the position and size of the square
rect = pygame.Rect(100, 100,200,200)
# Draw the square
pygame.draw.rect(screen, (0, 0, 0), rect, 1)
pygame.display.flip()
# Keep the window open until it is closed
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
Above is the code for drawing a square pygame module you can run this code on your machine or using an online python compiler and see the output.
Before running you need to install pygame module in your system since we are using it, you can install it by using below command.
pip install pygame
Now you can run the program and after running it will open a new window and you will see a square drawn like below.
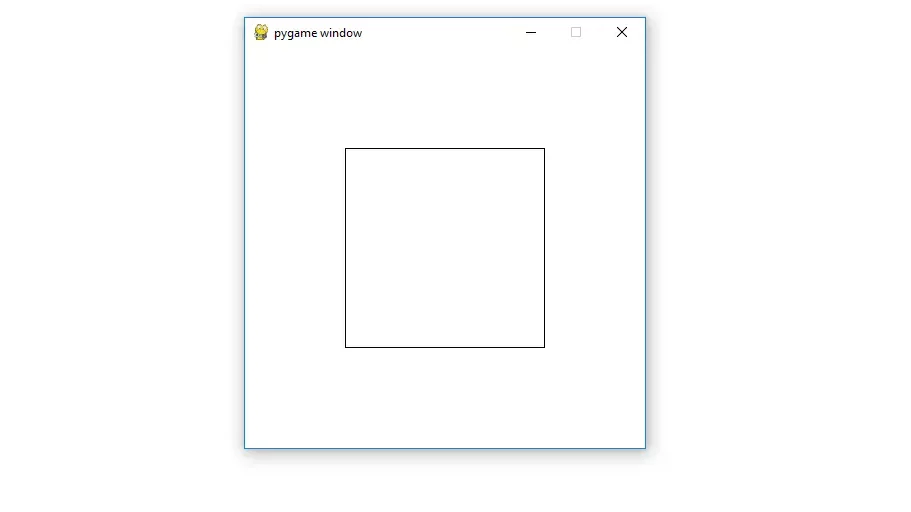
As you can see it successfully draws a square and we did not used turtle module, Now let’s see how to draw square using for loop.
Python Code For Drawing Square Using For Loop
# Set the side length of the square
side = 5
# Loop through each row
for i in range(side):
# Loop through each column in the row
for j in range(side):
# If we are on the first or last row, or the first or last column, print an asterisk
if i == 0 or i == side - 1 or j == 0 or j == side - 1:
print("*", end=" ")
# Otherwise, print a space
else:
print(" ", end=" ")
# Move to the next row
print()
Above is the code for drawing a square using for loop, you can run this code on your system or use this online python compiler, After running this program you will see a square pattern drawn like below.
* * * * *
* *
* *
* *
* * * * *
Want to draw a circle in python without using turtle refer here: Draw circle in python without turtle.
I hope you found this tutorial helpful and useful, Do share it with someone who might need this. If you want updates of our blog then join our telegram channel.
Thanks for reading, Have a nice day 🙂