Create Matrix Effect In Python With Code
Last updated June 6, 2023 by Jarvis Silva
In this python tutorial I will show you how to create a matrix effect in python programming, the matrix effect is just a bunch of 0 and 1 numbers printing in the terminal, It is also called the matrix rain effect.
If you are a beginner to python then don’t worry, I will show you step by step how to create the matrix rain effect in python and also provide you with the matrix rain code in python.
Matrix Effect Python Code
import os
import time
import random
os.system('color 0a')
nums = [1,0]
tm = 0
while tm < 30:
print(random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),)
tm = tm + 0.1
time.sleep(0.1)
Above is the matrix effect python code. First create a new folder on your computer for this project, open it in a code editor of your choice, create a python file and copy and paste the above python code in your file.
We have not used any external libraries for this project, so you don’t have to install anything. Now to run it you can use an online python compiler or run it on your computer.
I will recommend you to run it on your computer because it does not show the color in the online python compiler.
Don’t have python installed on your computer, you can refer to this guide to install python: Install and setup python on computer.
python filename.py
To run the program, open a command prompt or terminal at your project folder location and paste the above command to start the program.
The program will start and you will see that the console text is green and it goes on printing 0 and 1 creating a matrix effect, below is an example output of this program.
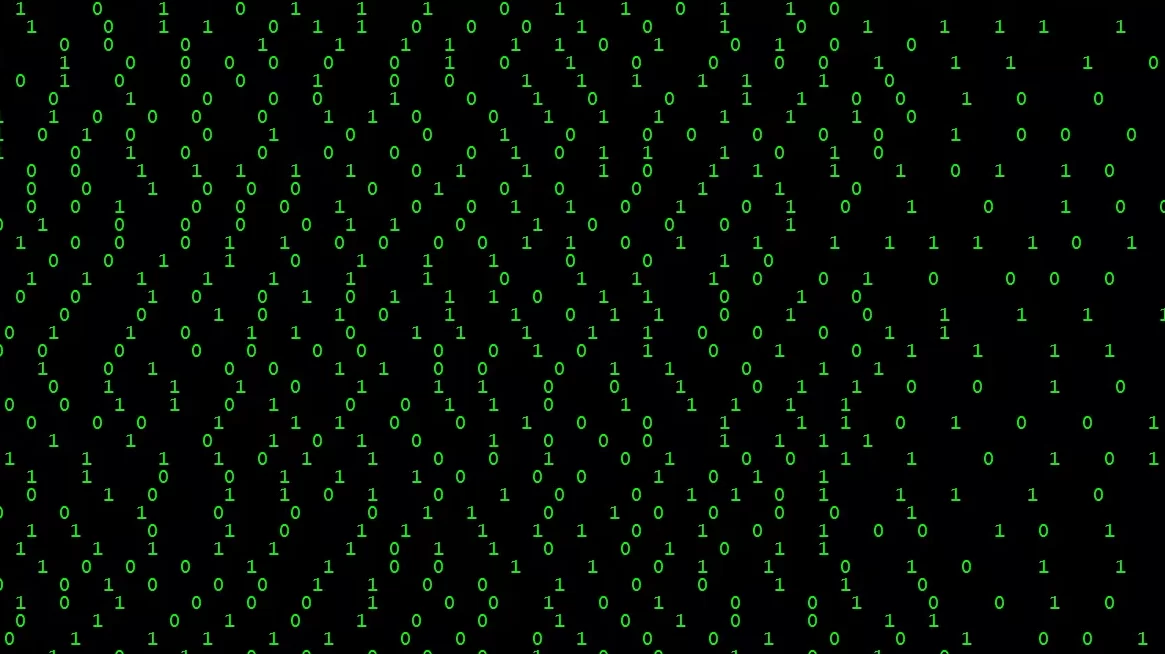
As you can see, we have successfully created the matrix effect in python programming. I hope you were able to run this program successfully.
Matrix Effect in Python Explanation
We have seen how to create a matrix effect using python in code. Now let’s see and understand how this python code work.
import os
import time
import random
First we import the required modules for this project which are installed with python setup so you don’t have to install them manually. Let’s see what each module does:
- os - It provides methods to interact with the operating system.
- time - You can use this to handle time related tasks.
- random - You can use this to generate random numbers.
Above are the only modules we need for this program, and now you know why we need them.
os.system('color 0a')
nums = [1,0]
Then we have these 2 lines of code. The first line is responsible to change the command prompt or terminal text to green.
Second line is a list with 1 and 0. These are the numbers which we want in the matrix effect. You can add any other number you want to add in the matrix effect.
tm = 0
while tm < 30:
print(random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),random.randrange(1,5)* " ",
random.choice(nums),)
Now we have a while loop which will run till tm variable is 30 inside the while loop. We print the matrix of 1 and 0 by using the below line.
print(random.randrange(1,5)* " ",random.choice(nums))
This line chooses a random number from the nums list and adds random space to it and prints it. You can first test it with just one statement; it will not cover the whole space in the terminal.
So I have printed it multiple times so it covers the whole area of the terminal, making it look like a matrix effect.
tm = tm + 0.1
time.sleep(0.1)
As you can see from the above code we increment the tm variable by 0.1, so the loop does not run infinitely, it will stop when it reaches 30.
Lastly, we use the time.sleep() function which pauses the program for 0.1 seconds which makes the matrix effect look more real.
Summary
This was the tutorial on how to create a matrix effect using python programming. I hope you found this tutorial helpful and useful. Do share it with your friends who might be interested in this python program.
Here are some more python guides you might find helpful:
- Vending Machine python program with code.
- NxNxN Matrix Python program With Code.
- Python program to check even odd numbers.
I hope you found what you were looking for from this tutorial. If you want more python tutorials like this, then do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂