Draw A Bird In Python Turtle With Code
Last updated June 6, 2023 by Jarvis Silva
In this tutorial we will see how to draw a bird in python, To create this program we will use the turtle module in python, It is a GUI python library which can be used to draw anything from characters, cartoons, shapes and other objects.
Python Code To Draw A Bird
import turtle
turtle.Screen().bgcolor("red")
tur = turtle.Turtle()
tur.pensize(7)
def go(x,y):
tur.penup()
tur.goto(x,y)
tur.pendown()
# Main Body
tur.fillcolor("#9cf71b")
go(-150,-70)
tur.seth(-30)
tur.begin_fill()
tur.circle(220,130)
tur.circle(120,180)
tur.circle(-120,110)
tur.seth(-52)
tur.circle(220,30)
tur.end_fill()
# Feather
tur.fillcolor("#dcf53b")
go(-100,90)
tur.seth(160)
tur.begin_fill()
tur.circle(-25,180)
tur.circle(-120,60)
tur.circle(-40,60)
tur.circle(-120,60)
tur.circle(-30,180)
tur.seth(180)
tur.circle(-50,45)
tur.circle(-30,190)
tur.end_fill()
# Eye
tur.fillcolor("#1c0508")
tur.pencolor("#1c0508")
tur.pensize(7)
go(60,200)
tur.seth(-180)
tur.begin_fill()
tur.circle(35)
tur.end_fill()
tur.fillcolor("white")
tur.begin_fill()
tur.circle(35,180)
tur.end_fill()
# Foot
go(-30,-100)
tur.seth(-80)
tur.pensize(10)
tur.circle(-90,40)
go(30,-90)
tur.seth(-80)
tur.pensize(10)
tur.circle(-120,35)
# Chonch
go(180,170)
tur.seth(15)
tur.circle(60,30)
go(180,160)
tur.seth(-15)
tur.circle(-50,40)
tur.hideturtle()
turtle.done()
Above is the python program to draw a bird, the whole program is made using turtle functions so let’s see how this code works:
- The code starts by importing the turtle module, which provides a way to create graphics using a turtle object.
- The turtle.Screen() function creates a canvas/screen with a red background color.
- A turtle object named ‘tur’ is created, and its pen size is set to 7.
- The ‘go’ function is defined, which is used to move the turtle object to a specific position on the canvas.
- The main body of the bird is drawn using a combination of circles and arcs. The fill color is set to a greenish color (#9cf71b).
- The feather on the bird’s head is drawn using another combination of circles and arcs. The fill color is set to a yellowish color (#dcf53b).
- The bird’s eye is drawn as a filled circle with a black border. The fill color is set to a dark color (#1c0508), and the pen color is also set to the same color.
- The bird’s foot is drawn using two sets of circles and arcs. The pen size is set to 10 for drawing thicker lines.
- The code ends by hiding the turtle object and calling turtle.done() to finish the drawing.
You understood this code now before running it you might need to install the turtle module mostly it comes pre-installed with python but if it is not then use below command to install.
pip install turtle
Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or else you can use this online python compiler.
When you run the program and it will open a new window and it will start drawing a bird and below is the finished drawing of a simple cute bird.
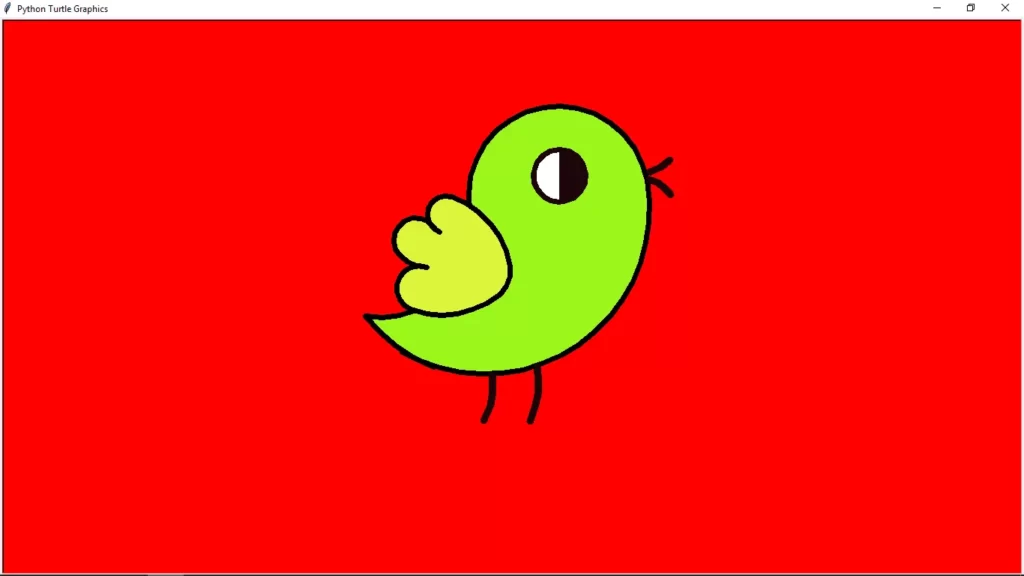
As you can see, we successfully drew a bird using python turtle, I hope you found this tutorial helpful and useful, do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
- Draw Panda In Python Turtle With Code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂