Draw Chess Board In Python Turtle
Published February 2, 2024 by Jarvis Silva
In this tutorial I will show you how to draw chess board in python using the built in turtle module so follow along till the end.
Turtle module in python provides us with the tools to create graphics, illustrations and more so now let’s draw a chess board.
Python Code To Draw A Chess Board
import turtle
# create screen object
sc = turtle.Screen()
# create turtle object
pen = turtle.Turtle()
# method to draw square
def draw():
for i in range(4):
pen.forward(30)
pen.left(90)
pen.forward(30)
# Driver Code
if __name__ == "__main__" :
# set screen
sc.setup(600, 600)
# set turtle object speed
pen.speed(100)
# loops for board
for i in range(8):
# not ready to draw
pen.up()
# set position for every row
pen.setpos(0, 30 * i)
# ready to draw
pen.down()
# row
for j in range(8):
# conditions for alternative color
if (i + j)% 2 == 0:
col ='black'
else:
col ='white'
# fill with given color
pen.fillcolor(col)
# start filling with colour
pen.begin_fill()
# call method
draw()
# stop filling
pen.end_fill()
# hide the turtle
pen.hideturtle()
turtle.done()
Output
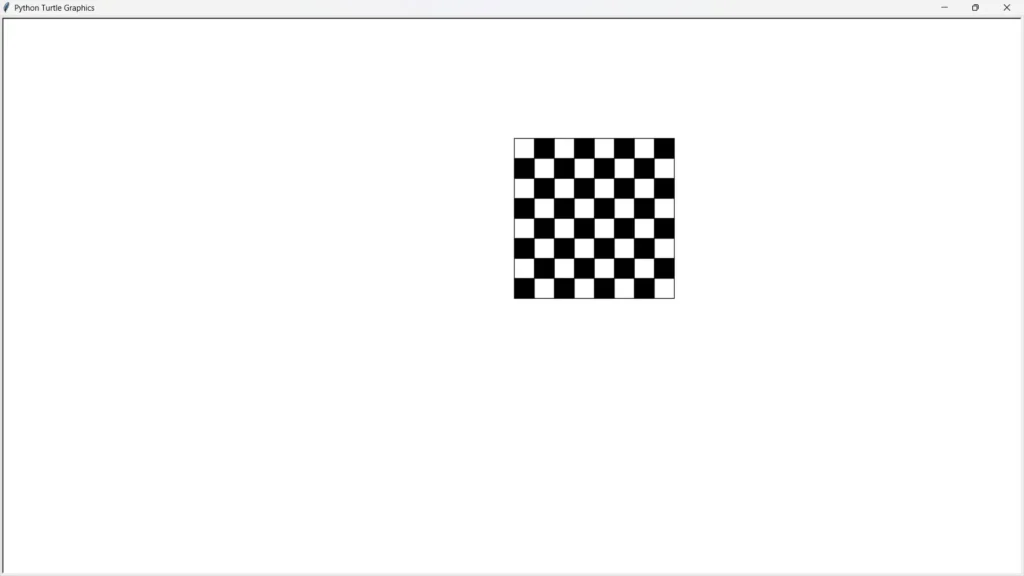
So as you can see we successfully drawn a chess board using python, I hope you were able to follow along and create this program.
Also Draw Lion In Python Using Turtle Graphics.
Thank you for reading, Have a nice day 🙂
Related content
View All
Draw Luffy Using Python With Code >
Draw Lion In Python Using Turtle Graphics >
Draw A Rhombus In Python Turtle >
Draw Lord Shiva Using Python >
Python Program To Draw Sharingan >
Draw Hexagon Using Python Turtle >
Python Turtle Program To Draw Four Circles >
Draw Minion Using Python Turtle >
Draw Middle Finger In Python >
Python Program To Draw Cindrella >
How To Draw a Half Circle In Python Turtle >
Draw Hanuman Ji Using Python >