Draw A Fish In Python Using Turtle
Last updated July 3, 2023 by Jarvis Silva
Want to know how to draw a fish using python then you are at the right place today in this tutorial, we will see how can we draw a fish using our python programming skills.
To create this program we will use the turtle module, It is a GUI python library which can be used to draw anything from characters, cartoons, shapes and other objects.
Python Code To Draw A Fish
import turtle
fish = turtle
fish.color('yellow')
fish.goto(0,0)
fish.speed(10)
fish.Screen().bgcolor("blue")
fish.left(45)
fish.forward(100)
fish.right(135)
fish.forward(130)
fish.right(130)
fish.forward(90)
fish.left(90)
fish.right(90)
fish.circle(200,90)
fish.left(90)
fish.circle(200,90)
fish.penup()
fish.left(130)
fish.forward(200)
fish.pendown()
fish.circle(10,360)
fish.right(270)
fish.penup()
fish.forward(50)
fish.pendown()
fish.left(90)
fish.circle(100,45)
fish.penup()
fish.forward(300)
fish.left(135)
fish.pendown()
fish.right(180)
fish.done()
Above is the code for drawing a fish, if you see in the code it uses all turtle functions so let’s see how they work:
import turtle
: Imports the Turtle module.fish = turtle
: Assigns the module to a variable namedfish
.fish.color('yellow')
: Sets the color of the turtle to yellow.fish.goto(0,0)
: Moves the turtle to the coordinates (0, 0) on the screen.fish.speed(10)
: Sets the speed of the turtle to 10.fish.Screen().bgcolor("blue")
: Sets the background color of the turtle screen to blue.- The following lines use various turtle commands to draw the shape of a fish:
fish.left(45)
: Turns the turtle left by 45 degrees.fish.forward(100)
: Moves the turtle forward by 100 units.fish.right(135)
: Turns the turtle right by 135 degrees.fish.forward(130)
: Moves the turtle forward by 130 units.fish.right(130)
: Turns the turtle right by 130 degrees.fish.forward(90)
: Moves the turtle forward by 90 units.fish.left(90)
: Turns the turtle left by 90 degrees.fish.right(90)
: Turns the turtle right by 90 degrees.fish.circle(200,90)
: Draws a quarter-circle with a radius of 200 units and an angle of 90 degrees.fish.left(90)
: Turns the turtle left by 90 degrees.fish.circle(200,90)
: Draws another quarter-circle with the same radius and angle.fish.penup()
: Lifts the turtle’s pen off the screen.fish.left(130)
: Turns the turtle left by 130 degrees.fish.forward(200)
: Moves the turtle forward by 200 units.fish.pendown()
: Puts the turtle’s pen back on the screen.fish.circle(10,360)
: Draws a small circle with a radius of 10 units.fish.right(270)
: Turns the turtle right by 270 degrees.fish.penup()
: Lifts the turtle’s pen off the screen.fish.forward(50)
: Moves the turtle forward by 50 units.fish.pendown()
: Puts the turtle’s pen back on the screen.fish.left(90)
: Turns the turtle left by 90 degrees.fish.circle(100,45)
: Draws a quarter-circle with a radius of 100 units and an angle of 45 degrees.fish.penup()
: Lifts the turtle’s pen off the screen.fish.forward(300)
: Moves the turtle forward by 300 units.fish.left(135)
: Turns the turtle left by 135 degrees.fish.pendown()
: Puts the turtle’s pen back on the screen.fish.right(180)
: Turns the turtle right by 180 degrees.
fish.done()
: Indicates that the turtle drawing is complete.
As we have used the turtle module we might need to install it before we run this program, mostly it comes preinstalled but if you get any errors like module not found then install it by using below command.
pip install turtle
You can now run this program on your computer for that you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or you can use this online python compiler.
After running this program it will open a new window and it will start drawing a fish and below is the finished drawing of a simple fish.
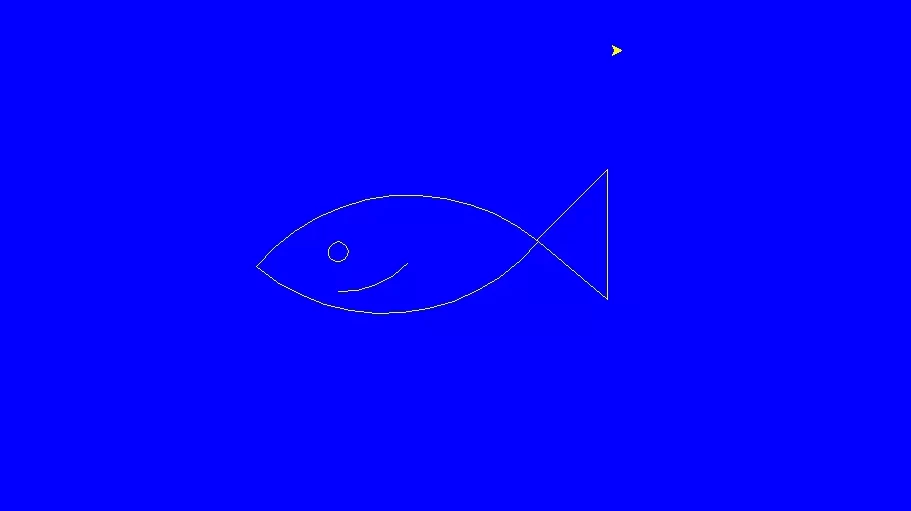
As you can see, we successfully drew a fish using python turtle, I hope you found this tutorial helpful and useful, do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
- Draw Panda In Python Turtle With Code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂