Draw Shivaji Maharaj In Python
Last updated July 3, 2023 by Jarvis Silva
Today in this tutorial we will see how to draw Shivaji Maharaj in python, Chhatrapati Shivaji Maharaj is one of the greatest indian warrior, He was a maratha king who established maratha empire in western india.
So to draw this great person we will use a python library called turtle which can be used to draw graphics in python so let’s start.
Python Code To Draw Shivaji Maharaj
import turtle as tur
tur.bgcolor("orangered")
tur.screensize(1400,1000)
corr = [(780,-209),(750,-208),(700,-207),(650,-206),
(580,-205),(560,-203),(540,-200),(520,-198),
(520,-193),(526,-190),(530,-180),(536,-172),
(538,-160),(543,-123),(540,-112),(533,-100),
(525,-86),(525,-80),(530,-60),(532,-40),
(531,-24),(534,-21),(540,-23),(550,-26),
(553,-30),(556,-40),(540,-90),(540,-108),
(543,-120),(550,-126),(567,-136),(562,-120),
(562,-115),(567,-100),(578,-80),(580,-76),
(584,-60),(586,-40),(582,-20),(580,-14),
(570,-9),(560,-5),(550,-6),(540,-8),
(530,-11),(518,0),(512,8),(502,8),(500,10),
(482,20),(470,36),(460,49),(468,63),(465,66),
(470,80),(468,100),(466,110),(460,120),
(458,123),(455,122),(452,130),(456,136),
(460,145),(463,133),(459,128),(472,128),
(468,140),(463,157),(470,157),(478,152),
(475,166),(471,165),(460,162),(457,165),
(443,165),(437,160),(427,153),(423,149),
(424,138),(419,133),(419,130),(422,128),
(423,127),(426,124),(423,120),(423,118),
(420,116),(420,114),(428,116),(430,118),
(432,118),(432,116),(428,114),(414,92),
(405,84),(390,77),(386,79),(377,79),
(375,76),(364,76),(357,88),(352,86),
(348,92),(340,93),(330,96),(326,96),
(320,92),(312,97),(307,92),(304,95),
(306,100),(308,102),(307,104),(307,108),
(304,107),(304,120),(303,124),(308,132),
(300,129),(298,128),(288,120),(282,104),
(283,100),(284,97),(288,92),(285,89),
(278,87),(283,77),(281,72),(288,67),
(287,59),(284,57),(284,54),(287,50),
(286,48),(290,43),(288,40),(300,26),
(298,17),(305,14),(312,15),(316,17),
(320,20),(322,40),(324,47),(326,49),
(333,40),(342,18),(343,3),(345,-6),
(342,-18),(342,-22),(346,-36),(350,-38),
(348,-43),(340,-41),(336,-37),(320,-33),
(312,-40),(307,-47),(303,-100),(308,-115),
(314,-118),(320,-120),(328,-122),(328,-110),
(324,-103),(318,-100),(319,-61),(322,-57),
(340,-60),(366,-78),(366,-100),(368,-106),
(366,-120),(363,-140),(361,-157),(358,-163),
(350,-166),(340,-178),(320,-164),(300,-151),
(280,-140),(260,-130),(240,-122),(220,-112),
(200,-102),(180,-100),(150,-98),(125,-96),
(100,-94),(75,-92),(50,-90),(25,-88),
(0,-86),(-25,-83),(-50,-81),(-75,-79),
(-100,-77),(-125,-75),(-150,-72),(-175,-70),
(-800,-68),
(-800,-500),(800,-500),(800,-210)]
part1 = [(418,70),(412,73),(404,67),(393,64),
(382,66),(382,60),(390,58),(392,50),
(400,46),(402,48),(408,51),(417,58),(418,60)]
part2 = [(380,-186),(400,-190),(420,-193),(440,-195),
(460,-197),(503,-199),(508,-186),(520,-174),
(520,-162),(528,-158),(531,-127),(520,-113),
(500,-106),(495,-102),(500,-117),(500,-123),
(480,-132),(452,-153),(440,-153),(440,-163),
(430,-177),(424,-180),(420,-176),(426,-160),
(437,-144),(440,-145),(467,-122),(467,-120),
(460,-107),(453,-100),(450,-85),(450,-72),
(440,-74),(408,-72),(400,-80),(397,-80),
(390,-77),(386,-85),(380,-108),(377,-123),
(375,-140),(370,-160),(374,-166),(362,-180)]
points = [[(-390,300),(-370,300),(-370,270),(-390,270),(-390,300)],
[(-250,300),(-230,300),(-230,270),(-250,270),(-250,300)],
[(-180,300),(-160,300),(-160,270),(-180,270),(-180,300)],
[(-110,300),(-90,300),(-90,270),(-110,270),(-110,300)],
[(-40,300),(-20,300),(-20,270),(-40,270),(-40,300)]]
def sun():
tur.penup()
tur.speed(5)
tur.goto(420,-220)
tur.pendown()
tur.color("gold")
tur.begin_fill()
tur.circle(240)
tur.end_fill()
def draw(c):
tur.penup()
tur.speed(7)
tur.goto(800,-210)
tur.pendown()
tur.color("black")
tur.begin_fill()
for i in range(len(c)):
x, y = c[i]
tur.goto(x, y)
tur.end_fill()
def part(p,g):
tur.penup()
tur.speed(6)
tur.goto(g)
tur.pendown()
tur.color("gold")
tur.begin_fill()
for i in range(len(p)):
x, y = p[i]
tur.goto(x, y)
tur.end_fill()
part1Goto = (417,60)
part2Goto = (362,-180)
tur.speed(15)
wn = tur.Screen()
wn.screensize()
wn.setup(width = 1.0, height = 1.0)
sun()
draw(corr)
part(part1,part1Goto)
part(part2,part2Goto)
tur.hideturtle()
tur.done()
Above is code for drawing the great Shivaji Maharaj so copy the code and paste it in a python file and run this program on your computer or don’t have python installed you can run it using this online python compiler.
As I have told you that we have used turtle library so we need to install it. It comes preinstalled with python but if you get any errors then you can use below command to install the library.
pip install turtle
After running this program you should see a beautiful drawing of the great Shivaji Maharaj on horse with sunset view below is the output of this program.
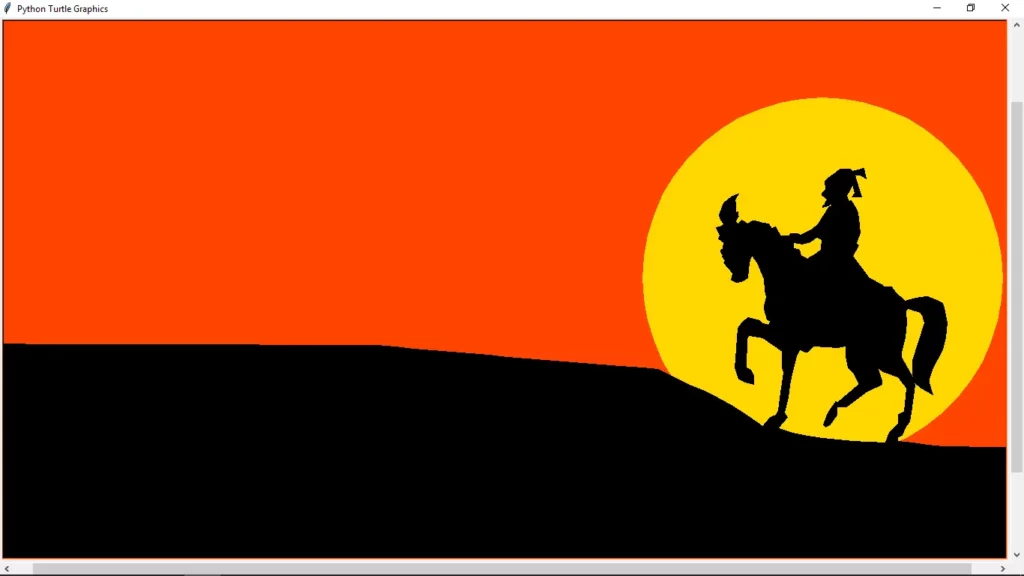
I hope you found this tutorial helpful, Do share it with your friends someone who is intrested in shivaji maharaj.
Here are some more drawing tutorials for you:
- Draw Mickey Mouse In Python Turtle.
- Awesome Python Turtle Drawing Codes.
- Draw Rainbow In Python Turtle.
Want more python tutorials like this then stay updated by joining our telegram channel where we post regular blog updates.
Thank you for reading, Have a nice day 🙂