Draw Superman Logo Using Python Turtle
Last updated July 3, 2023 by Jarvis Silva
Want to know how to draw Superman Logo using python then you are at the right place, Superman is one of the most popular superhero from the DC movies who is the most strongest superhero I have ever seen.
So inorder to draw it’s logo in python we will use the turtle module, It is a GUI module which can be used to draw anything in python from characters to logos.
Python Code To Draw Superman Logo
import turtle
t=turtle.Turtle()
turtle.Screen().bgcolor('#f3f3f3')
def curve(value): #create a function to generate curves in turtle
for i in range(value): #for loop to repeat the inputted value number of times
t.right(1) #step by step curve
t.forward(1)
t.penup() #pen is in the up position so it will not draw
t.setposition(0,43) #move the pen to these x and y coordinates
t.pendown() #pen is in the down position so it will draw
t.begin_fill() #start filling in the shape
t.pencolor('black') #change the pen color to black
t.fillcolor('red') #change the shape fill color to maroon to match the Superman logo
t.pensize(3) #use a pen size of 3 to create a black border
t.forward(81.5) #the pen will move forward this number to start the shield of the logo
t.right(49.4) #rotate the pen right 49.4 degrees
t.forward(58) #move the pen forward by 58
t.right(81.42) #rotate right by 81.42 degrees
t.forward(182) #move the pen forward by 182
t.right(98.36) #rotate the pen right by 98.36 degrees
t.forward(182) #move the pen forward by 182
t.right(81.42) #rotate the pen by 81.42 degrees to the right
t.forward(58) #move the pen forward 58
t.right(49.4) #rotate the pen to the right by 49.4
t.forward(81.5) #move the pen forward by 81.5 to meet the start at the top of the shield
t.end_fill() #complete the fill of the shield so the shape is closed off
t.penup() #pen will not draw
t.setposition(38,32) #now to create the yellow parts of the Superman logo
t.pendown() #the pen is now poised to draw
t.begin_fill() #start a new shape
t.fillcolor('gold') #change the fill color to gold to match the Superman logo
t.forward(13) #move the pen forward by 13
t.right(120) #rotate the pen right by 120 degrees
t.forward(13) #move the pen forward by 13
t.right(120) #rotate the pen right by 120 degrees
t.forward(13) #move the pen forward by 13
t.end_fill() #the small triangle on the right is now complete
t.penup() #stop the pen from drawing
t.setposition(81.5,25) #now to create the larger yellow part of the Superman logo, change the position of the pen
t.pendown() #the pen will now draw again
t.begin_fill() #the fill is still the same color set before
t.right(210) #rotate the pen right by 210 degrees
t.forward(25) #move the pen forward by 25
t.right(90) #rotate the pen right by 90 degrees
t.forward(38) #move the pen forward by 38
t.right(45) #rotate the pen right by 45 degrees
t.circle(82,90) #this function is used to draw a circle in turtle, the first integer is the radius and the second is the number of degrees of the circle drawn
t.left(90) #rotate the pen left by 90 degrees
t.circle(82,60) #create a circle of radius 82 and only complete 60 degrees of the circle
curve(61) #call the curve function that was previously defined, pass an integer value equal to the length of the curve desired
t.left(90) #rotate the pen left by 90 degrees
t.forward(57) #move the pen forward by 57
t.left(90) #rotate the pen left by 90 degrees
t.forward(32) #move the pen forward by 32
t.end_fill() #fill in the larger yellow part of the logo
t.penup() #stop drawing
t.home() #reset the pen location to the origin so the orientation is also reset
t.setposition(-69,-38) #finish the major parts of the S superimposition on the shield
t.pendown()
t.begin_fill()
curve(20)
t.forward(33)
t.left(10)
t.circle(82,20)
curve(30)
t.forward(10)
t.right(110)
curve(40)
t.right(10)
t.circle(50,10)
curve(45)
t.right(5)
t.forward(45)
t.end_fill()
t.penup()
t.home()
t.setposition(20,-100)
t.pendown()
t.begin_fill()
t.right(135)
t.forward(27)
t.right(90)
t.forward(27)
t.right(135)
t.forward(38.18)
t.end_fill()
t.penup()
t.home()
t.setposition(-57,32)
t.pendown()
t.begin_fill()
t.right(180)
t.forward(18)
t.left(45)
t.forward(44)
t.left(80)
t.forward(15)
t.left(130)
curve(40)
t.forward(20)
t.end_fill()
t.hideturtle()
turtle.exitonclick()
Above is the code to draw the Superman Logo, if you see in the code it is all turtle functions so let’s see how this code works.
- The turtle module is imported to create graphics using a turtle-like drawing board.
- The code defines a function called
curve
to generate curves in turtle. - The turtle’s position, pen color, fill color, and pen size are set for drawing the Superman logo.
- The turtle moves and turns to create the shield shape of the logo.
- The turtle creates smaller yellow triangles on the shield.
- The turtle creates a larger yellow part of the logo using circles and curves.
- The turtle creates the ‘S’ superimposed on the shield using various movements.
- The turtle creates additional shapes and patterns.
- The turtle is hidden from view.
- The program waits for a click on the screen to exit.
Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or else you can use this online python compiler.
Now you have the code, as we used the turtle library for this program so you might need to install it if you get any errors like turtle module not found, mostly it comes pre-installed with python setup, but if you get any errors use below command to install.
pip install turtle
So now you have everything setup and you are ready to run the program, so to run this program open a command prompt at your program folder location and paste the below command.
python filename.py
The above command will run the program and it will open a new window and it will start drawing the Superman Logo and below is the finished drawing of the logo.
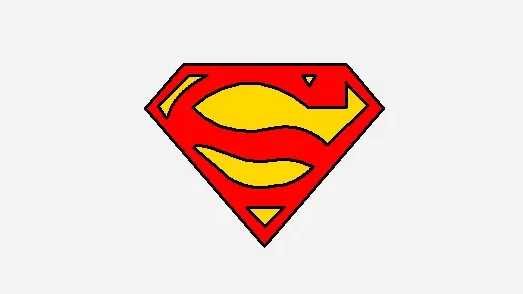
As you can see, we successfully drew the Superman Logo, I hope you found this tutorial helpful and useful, Do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
- Draw Panda In Python Turtle With Code.
- Draw Netflix Logo Using Python Turtle.
- Draw Whatsapp Logo Using Python Turtle.
- Draw Windows Logo Using Python.
- Draw Apple Logo Using Python Turtle.
- Draw YouTube Logo Using Python Turtle.
- Draw India Map In Python Turtle.
- Draw Snapchat Logo Using Python Turtle.
- Draw TikTok Logo Using Python.
- Draw Instagram Logo Using Python Turtle.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂