Draw American Flag In Python
Last updated July 3, 2023 by Jarvis Silva
Want to know how to draw american flag in python then you are at the right place, In this tutorial we will see how to draw the american flag using our python skills.
To draw usa flag in python, we will use the python turtle module, It is GUI module which can be used to draw things in python
Python Code To Draw American Flag
import turtle
import time
# create a screen
screen = turtle.getscreen()
# set background color of screen
screen.bgcolor("white")
# set tile of screen
screen.title("American Flag")
t = turtle.Turtle()
# set the cursor/turtle speed. Higher value, faster is the turtle
t.speed(100)
t.penup()
# decide the shape of cursor/turtle
t.shape("turtle")
# flag height to width ratio is 1:1.9
flag_height = 250
flag_width = 475
# starting points
# start from the first quardant, half of flag width and half of flag height
start_x = -237
start_y = 125
# For red and white stripes (total 13 stripes in flag), each strip width will be flag_height/13 = 19.2 approx
stripe_height = flag_height/13
stripe_width = flag_width
# length of one arm of star
star_size = 10
def draw_fill_rectangle(x, y, height, width, color):
t.goto(x,y)
t.pendown()
t.color(color)
t.begin_fill()
t.forward(width)
t.right(90)
t.forward(height)
t.right(90)
t.forward(width)
t.right(90)
t.forward(height)
t.right(90)
t.end_fill()
t.penup()
def draw_star(x,y,color,length) :
t.goto(x,y)
t.setheading(0)
t.pendown()
t.begin_fill()
t.color(color)
for turn in range(0,5) :
t.forward(length)
t.right(144)
t.forward(length)
t.right(144)
t.end_fill()
t.penup()
# this function is used to create 13 red and white stripes of flag
def draw_stripes():
x = start_x
y = start_y
# we need to draw total 13 stripes, 7 red and 6 white
# so we first create, 6 red and 6 white stripes alternatively
for stripe in range(0,6):
for color in ["red", "white"]:
draw_fill_rectangle(x, y, stripe_height, stripe_width, color)
# decrease value of y by stripe_height
y = y - stripe_height
# create last red stripe
draw_fill_rectangle(x, y, stripe_height, stripe_width, 'red')
y = y - stripe_height
# this function create navy color square
# height = 7/13 of flag_height
# width = 0.76 * flag_height
# check references section for these values
def draw_square():
square_height = (7/13) * flag_height
square_width = (0.76) * flag_height
draw_fill_rectangle(start_x, start_y, square_height, square_width, 'navy')
def draw_six_stars_rows():
gap_between_stars = 30
gap_between_lines = stripe_height + 6
y = 112
# create 5 rows of stars
for row in range(0,5) :
x = -222
# create 6 stars in each row
for star in range (0,6) :
draw_star(x, y, 'white', star_size)
x = x + gap_between_stars
y = y - gap_between_lines
def draw_five_stars_rows():
gap_between_stars = 30
gap_between_lines = stripe_height + 6
y = 100
# create 4 rows of stars
for row in range(0,4) :
x = -206
# create 5 stars in each row
for star in range (0,5) :
draw_star(x, y, 'white', star_size)
x = x + gap_between_stars
y = y - gap_between_lines
# start after 5 seconds.
time.sleep(5)
# draw 13 stripes
draw_stripes()
# draw squares to hold stars
draw_square()
# draw 30 stars, 6 * 5
draw_six_stars_rows()
# draw 20 stars, 5 * 4. total 50 stars representing 50 states of USA
draw_five_stars_rows()
# hide the cursor/turtle
t.hideturtle()
# keep holding the screen until closed manually
screen.mainloop()
Above is the code to draw the american flag, as you can see it is a long code so below are some points on how this program works.
- It sets the background color of the screen to white and the title to “American Flag.”
- It initializes a turtle object named ‘t’.
- It sets the speed of the turtle to 100 (higher value means faster drawing).
- It defines a function called draw_fill_rectangle() to draw filled rectangles with the given dimensions and color.
- It defines a function called draw_star() to draw a star at the specified position with the given color and size.
- It initializes various variables and constants related to the flag’s dimensions and sizes.
- It defines a function called draw_stripes() to draw the 13 red and white stripes of the flag.
- It defines a function called draw_square() to draw the navy-colored square on the flag for the stars.
- It defines a function called draw_six_stars_rows() to draw the rows of stars on the flag (5 rows with 6 stars each).
- It defines a function called draw_five_stars_rows() to draw the rows of stars on the flag (4 rows with 5 stars each).
- It pauses the execution for 5 seconds using the time.sleep() function.
- It calls the draw_stripes() function to draw the stripes on the flag.
- It calls the draw_square() function to draw the navy-colored square on the flag.
- It calls the draw_six_stars_rows() function to draw the rows of stars (6 stars per row) on the flag.
- It calls the draw_five_stars_rows() function to draw the rows of stars (5 stars per row) on the flag.
Now to run this program you can use this online python compiler or run it on your machine, to run on your machine you might need to install the turtle module you can do that by using below command
pip install turtle
It will install the module on your computer, Now you can run the program on your computer, it will open a new window and it will start drawing the american flag, below is the output you should get.
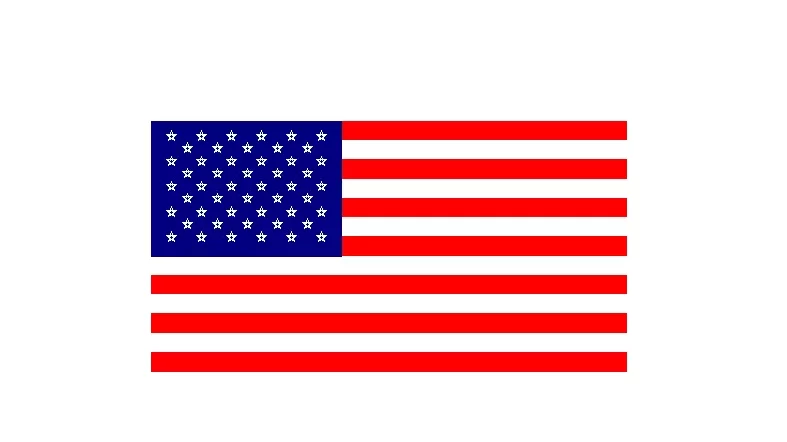
As you can see, It successfully drew the american flag so this was the tutorial on how to draw american flag in python, I hope you found this python tutorial helpful and useful. Do share this guide with your friends or anyone who needs it.
Here are more python drawing tutorials for you:
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Captain America shield in python.
I hope you found what you were looking for from this python tutorial and If you want more python tutorials like this do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂