Draw A Dog In Python
Last updated July 3, 2023 by Jarvis Silva
In this tutorial I will show you how to draw dog in python, to draw dog we will use the turtle module which allows us to draw graphics in python.
Python Code To Draw Dog
import turtle as t
t.screensize(500, 500)
# 【 head outline 】
t.pensize(5)
t.home()
t.seth(0)
t.pd() #pendown
t.color('black')
t.circle(20, 80) # 0
t.circle(200, 30) # 1
t.circle(30, 60) # 2
t.circle(200, 29.5) # 3
t.color('black')
t.circle(20, 60) # 4
t.circle(-150, 22) # 5
t.circle(-50, 10) # 6
t.circle(50, 70) # 7
# determine the approximate position of the nose t.xcor and t. ycor the position of the tortoise at the beginning
x_nose = t.xcor()
y_nose = t.ycor()
t.circle(30, 62) # 8
t.circle(200, 15) # 9
# 【 nose 】
t.pu() #penup
t.goto(x_nose, y_nose + 25)
t.seth(90)
t.pd()
t.begin_fill()
t.circle(8)
t.end_fill()
# 【 eye 】
t.pu()
t.goto(x_nose + 48, y_nose + 55)
t.seth(90)
t.pd()
t.begin_fill()
t.circle(8)
t.end_fill()
# 【 ear 】
t.pu()
t.color('#444444')
t.goto(x_nose + 100, y_nose + 110)
t.seth(182)
t.pd()
t.circle(15, 45)
t.color('black')
t.circle(10, 15)
t.circle(90, 70)
t.circle(25, 110)
t.rt(4)
t.circle(90, 70)
t.circle(10, 15)
t.color('#444444')
t.circle(15, 45)
# 【 body 】
t.pu()
t.color('black')
t.goto(x_nose + 90, y_nose - 30)
t.seth(-130)
t.pd()
t.circle(250, 28)
t.circle(10, 140)
t.circle(-250, 25)
t.circle(-200, 25)
t.circle(-50, 85)
t.circle(8, 145)
t.circle(90, 45)
t.circle(550, 5)
# 【 tail 】
t.seth(0)
t.circle(60, 85)
t.circle(40, 65)
t.circle(40, 60)
t.lt(150) #left
t.circle(-40, 90)
t.circle(-25, 100)
t.lt(5)
t.fd(20)
t.circle(10, 60)
# 【 back 】
t.rt(80) #right
t.circle(200, 35)
# 【 collar 】
t.pensize(20)
t.color('#F03C3F')
t.lt(10)
t.circle(-200, 25)
# 【 love bell 】
t.pu()
t.fd(18)
t.lt(90)
t.fd(18)
t.pensize(6)
t.seth(35) #setheading
t.color('#FDAF17')
t.begin_fill()
t.lt(135)
t.fd(6)
t.right(180) # brush turn around
t.circle(6, -180)
t.backward(8)
t.right(90)
t.forward(6)
t.circle(-6, 180)
t.fd(15)
t.end_fill()
# 【 front calf 】
t.pensize(5)
t.pu()
t.color('black')
t.goto(x_nose + 100, y_nose - 125)
t.pd()
t.seth(-50)
t.fd(25)
t.circle(10, 150)
t.fd(25)
# 【 posterior calf 】
t.pensize(4)
t.pu()
t.goto(x_nose + 314, y_nose - 125)
t.pd()
t.seth(-95)
t.fd(25)
t.circle(-5, 150)
t.fd(2)
t.hideturtle()
t.done()
Above is the python code for drawing a dog, now let’s see how this program actually works in simple points:
- The turtle screen size is set to 500×500 pixels using
t.screensize(500, 500)
. - The code defines various functions and configurations for the turtle graphics.
- The outline of the head, including the mouth, nose, eyes, and ears, is drawn using a combination of circles and lines.
- The nose is drawn separately by moving the turtle to the appropriate position and drawing a filled circle.
- The eyes are drawn by moving the turtle to the correct position and drawing filled circles.
- The ears are drawn using a combination of circles and lines, with different colors applied.
- The body of the dog is drawn by moving the turtle to the correct position and drawing a series of circles and lines.
- The tail is drawn by moving the turtle in a specific pattern.
- The back of the dog is drawn using circles and lines.
- A collar is drawn around the neck using a thicker pen and a specific pattern.
- A love bell is drawn by moving the turtle to the correct position and drawing a pattern with a filled shape.
- The front and posterior calves are drawn by moving the turtle to the correct positions and drawing lines and circles.
- Finally, the turtle screen is closed using
t.done()
.
Now you have the code to run it you need to do one last thing as I have said I have used the turtle library for this program, so you might need to install it if you get any errors like turtle module not found to install use below command
pip install turtle
Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or else use this online python compiler.
When you run the program it will open a new window and it will start drawing the dog and below is the finished drawing of a fog
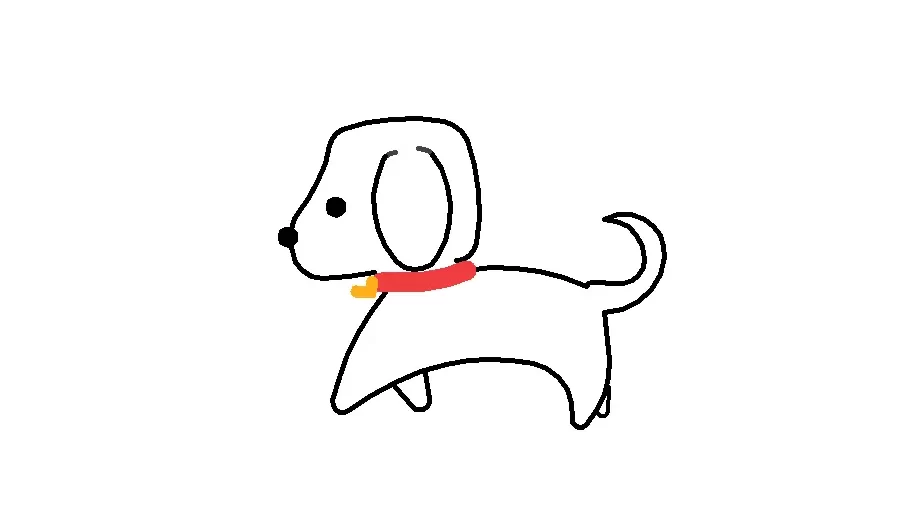
As you can see, we successfully drew a dog using our python skills, I know it is not a perfect drawing of a dog but we will improve upon this program in the future.
Here are some more python drawing tutorial for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo using python turtle.
- Draw Iron Man in python turtle with code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂