Draw Cat In Python Turtle
Last updated July 3, 2023 by Jarvis Silva
In this tutorial, I will show you how to draw a cat in python, to draw it we will use the turtle module, It is a GUI python library which can be used to draw anything from characters, cartoons, shapes and other objects.
Python Code To Draw A Cat
import turtle
import math
window = turtle.Screen()
window.bgcolor("yellow")
cursor = turtle.Turtle() # Cursor
cursor.shape("turtle")
cursor.color("black")
cursor.speed(3)
cursor.pensize(10)
def movePen(cursor, x, y):
cursor.penup()
cursor.setposition(x, y)
cursor.pendown()
def movePenX(cursor, x):
cursor.penup()
cursor.setx(x)
cursor.pendown()
def movePenY(cursor, y):
cursor.penup()
cursor.sety(y)
cursor.pendown()
def positionAlongCircle(x, y, radius, angle):
radians = math.radians(angle)
return [x + (radius*math.sin(radians)),
y + (radius*math.cos(radians))]
# Draw the head
movePenY(cursor, -150)
cursor.circle(150)
# Draw the nose
noseMouthOffset = -15
movePenY(cursor, -20 + noseMouthOffset)
cursor.circle(20)
# Draw the mouth
movePen(cursor, -100, -20 + noseMouthOffset)
cursor.right(90)
cursor.circle(50, 180)
cursor.left(180)
cursor.circle(50, 180)
# Draw the eyes
eyeSpacingX = 30
eyePosY = 40
eyeRadius = 30
# Right eye
movePen(cursor, eyeSpacingX, eyePosY)
cursor.right(180)
cursor.circle(eyeRadius, -180)
# Left eye
movePen(cursor, -eyeSpacingX, eyePosY)
cursor.circle(eyeRadius, 180)
# Draw the tongue
movePen(cursor, -20, -60 + noseMouthOffset)
cursor.circle(20, 180)
# Draw the ears
# Right ear
earBeginAngle = 25
earSize = 85
earWidth = 22
positionA = positionAlongCircle(0, 0, 150, earBeginAngle)
movePen(cursor, positionA[0], positionA[1])
positionB = positionAlongCircle(0, 0, 150 + earSize, earBeginAngle + earWidth)
cursor.setposition(positionB[0], positionB[1])
positionC = positionAlongCircle(0, 0, 150, earBeginAngle + earWidth * 2)
cursor.setposition(positionC[0], positionC[1])
# Left ear
positionA = positionAlongCircle(0, 0, 150, -earBeginAngle)
movePen(cursor, positionA[0], positionA[1])
positionB = positionAlongCircle(0, 0, 150 + earSize, -earBeginAngle + -earWidth)
cursor.setposition(positionB[0], positionB[1])
positionC = positionAlongCircle(0, 0, 150, -earBeginAngle + -earWidth * 2)
cursor.setposition(positionC[0], positionC[1])
# Whiskers
whiskerLength = 180
# Right whiskers
movePen(cursor, 50, -15)
cursor.setheading(0)
cursor.forward(whiskerLength)
movePen(cursor, 50, 0)
cursor.left(5)
cursor.forward(whiskerLength)
# Left whiskers
movePen(cursor, -50, -15)
cursor.setheading(180)
cursor.forward(whiskerLength)
movePen(cursor, -50, 0)
cursor.left(-5)
cursor.forward(whiskerLength)
window.exitonclick()
Above is the program for drawing a cat, in the code we use turtle functions to create this program so let’s how the code works:
- The code sets up the turtle window with a yellow background.
- A turtle named “cursor” is created with specific attributes (shape, color, speed, pensize).
- Several helper functions are defined to move the cursor to specific positions on the screen.
- The code draws the head of the smiley face by moving the cursor and drawing a circle.
- The code draws the nose by moving the cursor and drawing a smaller circle.
- The code draws the mouth by moving the cursor, rotating it, and drawing a semicircle.
- The code draws the eyes by moving the cursor and drawing semicircles in opposite directions.
- The code draws the tongue by moving the cursor and drawing a semicircle.
- The code draws the ears by calculating the positions along a circle and connecting them with lines.
- The code draws the whiskers by moving the cursor and drawing lines in different directions.
- The turtle window is set to close when the user clicks on it.
Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer.
Whe you run the program it will open a new window and it will start drawing a cat and below is the finished drawing of a cat.
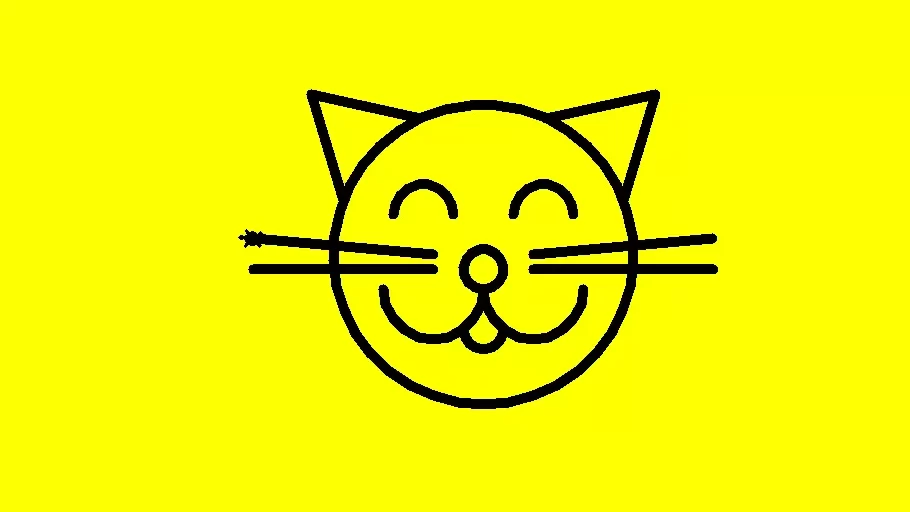
As you can see it successfully drew a cat using python turtle, I hope you were able to run this program successfully.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂