Draw Windows Logo Using Python
Last updated July 3, 2023 by Jarvis Silva
In this tutorial we will draw the Windows logo using python, windows is one of the most popular operating system used in most of the computers today.
We will use the turtle module to create this program in python, turtle is a GUI library with the help of it we can draw anything in python.
Python Code To Draw Windows Logo
from turtle import *
speed(1)
bgcolor('black')
penup()
goto(-50,60)
pendown()
color('blue')
begin_fill()
goto (100,100)
goto (100,-100)
#Draw windows
goto(-50,-60)
goto(-50,60)
end_fill()
color('black')
goto(15,100)
#cut 2 equal parts
color('black')
width(10)
goto (15,-100)
penup()
goto(100,0)
pendown()
goto(-100,0)
done()
Above is the code to draw the Windows logo. If you see in the code that it uses turtle functions so let’s see how this functions work:
- The code imports all functions from the Turtle module.
speed(1)
sets the drawing speed to 1, making it slower.bgcolor('black')
sets the background color of the drawing area to black.penup()
lifts the pen off the screen.goto(-50,60)
moves the turtle to the coordinates (-50, 60) without drawing.pendown()
puts the pen back on the screen.color('blue')
sets the pen color to blue.begin_fill()
indicates the start of a shape to be filled.goto (100,100)
moves the turtle to the coordinates (100, 100) and draws a line.goto (100,-100)
moves the turtle to the coordinates (100, -100) and draws a line.goto(-50,-60)
moves the turtle to the coordinates (-50, -60) and draws a line.goto(-50,60)
moves the turtle back to the initial position, completing the shape to be filled.end_fill()
fills the shape with the pen color, creating a solid blue figure.color('black')
changes the pen color to black.goto(15,100)
moves the turtle to the coordinates (15, 100) and draws a line.color('black')
sets the pen color to black again.width(10)
sets the pen width to 10.goto (15,-100)
moves the turtle to the coordinates (15, -100) and draws a line.penup()
lifts the pen off the screen.goto(100,0)
moves the turtle to the coordinates (100, 0) without drawing.pendown()
puts the pen back on the screen.goto(-100,0)
moves the turtle to the coordinates (-100, 0) and draws a line, cutting the figure into two equal parts.done()
signals the end of the drawing process.
Before you can run this program you might need to install turtle module if it is not pre-installed, you can install by using below command.
pip install turtle
Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or else you can use this online python compiler.
After running this program it will open a new window and it will start drawing the Windows Logo and below is the finished drawing of the logo.
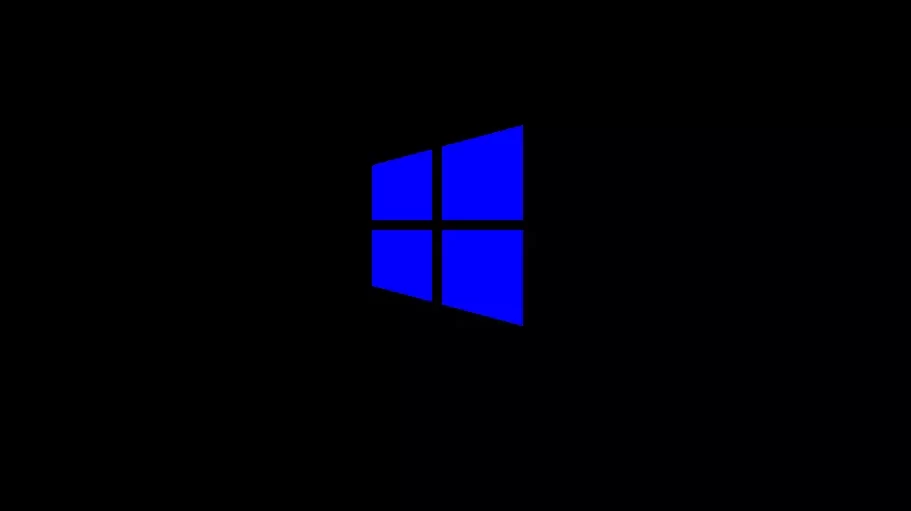
As you can see we successfully drew the Windows logo using python turtle, I hope you found this tutorial helpful and useful, Do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
- Draw Panda In Python Turtle With Code.
- Draw Netflix Logo Using Python Turtle.
- Draw Whatsapp Logo Using Python Turtle.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂