Draw Captain America Shield Using Python
Last updated July 3, 2023 by Jarvis Silva
Looking for a tutorial on how to draw captain america shield using python then you are at the right tutorial, today we will see how to draw the famous captain america shield using our python skills.
Captain America is one of the most popular character in the marvel universe, I am a marvel fan and I watched each and every marvel movie, so I decided to create this program.
To create this program we will need to use the turtle library which is a GUI library and can be used to draw things like characters or objects in python.
Python Code To Draw Captain America Shield
import turtle
import math
# Create a turtle object
ca = turtle.Turtle()
def func_1(x, y):
"""Move the turtle to the given coordinates and set up initial configuration"""
ca.penup()
ca.goto(x, y)
ca.pendown()
ca.setheading(0)
ca.pensize(2)
ca.speed(10)
def circle(r, color):
"""Draw a circle of the given radius and color"""
x_point = 0
y_pont = -r
func_1(x_point, y_pont)
ca.pencolor(color)
ca.fillcolor(color)
ca.begin_fill()
ca.circle(r)
ca.end_fill()
def star(r, color):
"""Draw a star of the given radius and color"""
func_1(0, 0)
ca.pencolor(color)
ca.setheading(162)
ca.forward(r)
ca.setheading(0)
ca.fillcolor(color)
ca.begin_fill()
for i in range(5):
ca.forward(math.cos(math.radians(18)) * 2 * r) # 2cos18°*r
ca.right(144)
ca.end_fill()
ca.hideturtle()
if __name__ == '__main__':
# Draw concentric circles with different colors
circle(288, 'crimson')
circle(234, 'snow')
circle(174, 'crimson')
circle(114, 'blue')
# Draw a star inside the innermost circle
star(114, 'snow')
# Finish drawing and keep the window open
turtle.done()
Above is the code for drawing the captain america shield, below is the explaination of how this code works
- The code imports the required modules,
turtle
andmath
. - It creates a turtle object named
ca
. - The
func_1
function moves the turtle to the given coordinates(x, y)
and sets up the initial configuration for drawing. - The
circle
function draws a circle with the given radiusr
and color. - The
star
function draws a star with the given radiusr
and color. - The
if __name__ == '__main__':
block is the entry point of the program. - Inside the
if
block, thecircle
function is called multiple times with different radius and color combinations to draw concentric circles. - After drawing the circles, the
star
function is called to draw a star inside the innermost circle. - Finally, the
turtle.done()
function is called to finish drawing and keep the turtle window open.
Now before you go and run this program if you get any errors like turtle module not found then you might need to install the turtle library as we have used it, mostly it comes preinstalled but still if you get errors use below command to install.
pip install turtle
The above command will install the python turtle module, If you don’t have python installed or setup, then you can refer to this article: Install and setup python or you can use this online python compiler.
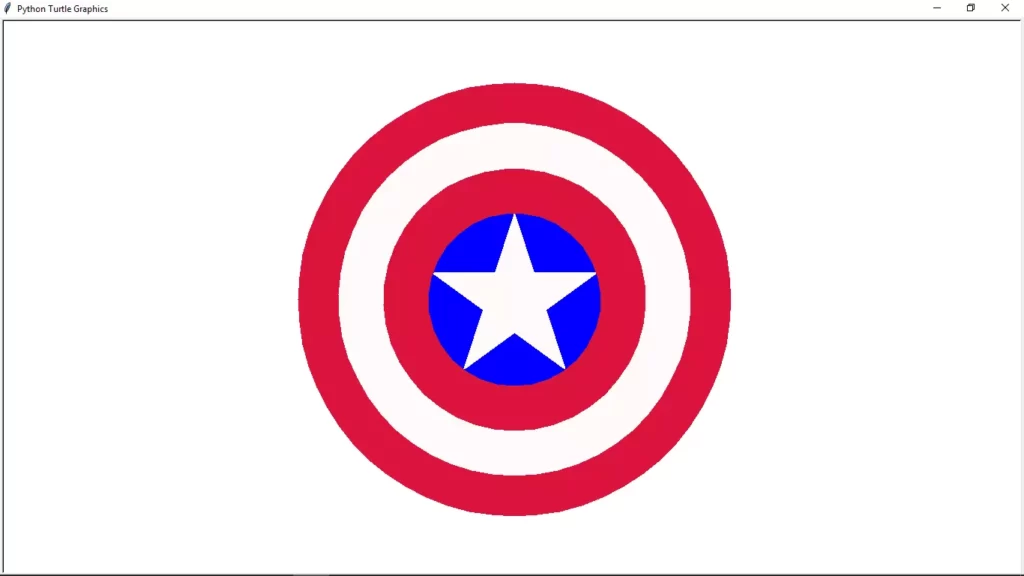
That above my friend is the captain america shield drawn using python turtle, it looks cool doesn’t it?
This was for the tutorial I hope you found this tutorial interesting and useful, do share this tutorial with your friends and family if they watch marvel movies they are definitely going to like this.
Here are more guides about drawing in python:
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
I hope you found what you were looking for and if you want more python drawing tutorials like this do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂