Draw Pubg Logo Using Python Turtle Library
Last updated July 3, 2023 by Jarvis Silva
In this tutorial we will see how to draw pubg logo using python, pubg is one of the most popular game, It is a multiplayer online battleground game I used to play it all day so today I have decided to create a tutorial on drawing its logo in python.
We will use the turtle module to create this program in python, turtle is a GUI library with the help of this library you can draw anything in python.
Python Code To Draw Pubg Logo
import turtle
t = turtle.Turtle()
turtle.bgcolor("black")
t.color("white")
def rect():
t.pensize(9)
t.forward(170)
t.left(45)
t.forward(6)
t.left(45)
t.forward(170)
t.left(45)
t.forward(6)
t.left(45)
t.forward(330)
t.left(45)
t.forward(6)
t.left(45)
t.forward(170)
t.left(45)
t.forward(6)
t.left(45)
t.forward(170)
def four_corner_lines():
t.pensize(12)
t.penup()
t.forward(180)
t.left(90)
t.forward(35)
t.left(90)
t.pendown()
t.forward(12)
t.penup()
t.forward(344)
t.pendown()
t.forward(17)
t.penup()
t.right(90)
t.forward(105)
t.right(90)
t.pendown()
t.forward(17)
t.penup()
t.forward(344)
t.pendown()
t.forward(12)
def p():
t.penup()
t.left(180)
t.forward(280)
t.pendown()
t.forward(40)
t.left(90)
t.forward(100)
t.left(180)
t.forward(52)
t.right(90)
t.forward(40)
t.left(90)
t.forward(47)
def u():
t.penup()
t.right(90)
t.forward(32)
t.right(90)
t.pendown()
t.forward(98)
t.left(90)
t.forward(40)
t.left(90)
t.forward(98)
def b():
t.penup()
t.right(90)
t.forward(35)
t.pendown()
t.forward(45)
t.right(90)
t.forward(43)
t.right(45)
t.forward(5)
t.right(45)
t.forward(40)
t.left(90)
t.forward(5)
t.left(90)
t.forward(40)
t.right(45)
t.forward(5)
t.right(45)
t.forward(40)
t.right(90)
t.forward(45)
t.right(90)
t.forward(96)
def g():
t.penup()
t.right(180)
t.forward(53)
t.left(90)
t.forward(98)
t.pendown()
t.forward(25)
t.right(90)
t.forward(45)
t.right(90)
t.forward(45)
t.right(90)
t.forward(95)
t.right(90)
t.forward(40)
rect()
four_corner_lines()
p()
u()
b()
g()
turtle.done()
Above is the code for draw the Pubg logo, as you can see in the code there are lot of turtle functions so let’s see how this code works:
- The code imports the
turtle
module, which allows for creating graphics and animations using a turtle-like object. - A turtle object
t
is created, which will be used to draw on the screen. - The background color is set to black and the turtle’s color is set to white.
- Several functions are defined to draw different parts of a graphical design.
- The
rect()
function is responsible for drawing a rectangular shape using a series of turtle movements and turns. - The
four_corner_lines()
function draws four lines at the corners of the rectangular shape. - The functions
p()
,u()
,b()
, andg()
draw specific letters by combining turtle movements and turns. - Finally, the functions are called in a specific order to create the desired graphical design.
- The
turtle.done()
function is called to indicate that the drawing is complete and to keep the turtle graphics window open until it is manually closed.
Above is a basic simple explaination of the code now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or you can use this online python compiler.
After you run the program it will open a new window and it will start drawing the Pubg Logo and below is the finished drawing of the logo.
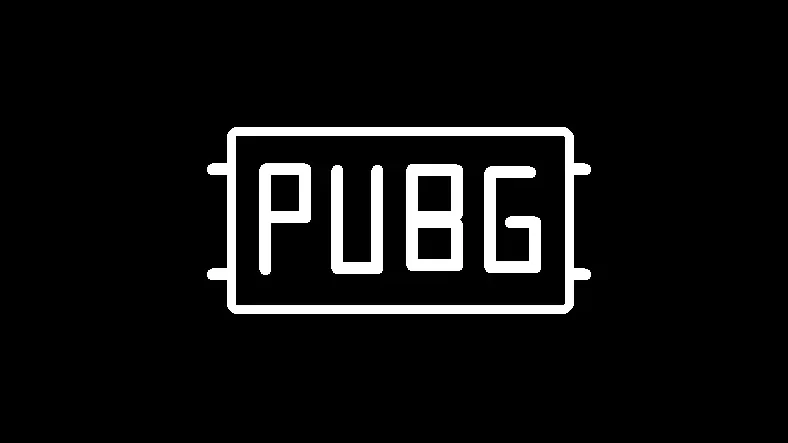
As you can see, we successfully drew the exact Pubg logo using python, I hope you found this tutorial helpful and useful, Do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
- Draw Panda In Python Turtle With Code.
- Draw Netflix Logo Using Python Turtle.
- Draw Whatsapp Logo Using Python Turtle.
- Draw Windows Logo Using Python.
- Draw Apple Logo Using Python Turtle.
- Draw YouTube Logo Using Python Turtle.
- Draw India Map In Python Turtle.
- Draw Snapchat Logo Using Python Turtle.
- Draw TikTok Logo Using Python.
- Draw Instagram Logo Using Python Turtle.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂