Draw A House Using Python Turtle
Last updated July 3, 2023 by Jarvis Silva
Want to draw a house using python then you are at the right place, in this tutorial we will see how to draw a simple house using our python skills.
We will use the turtle library in python to draw the house, Turtle module allows us to draw graphics in python if you are not familiar with turtle then don’t worry you can still follow along.
If you want more turtle drawing related tutorials then can go here python turtle drawing programs.
Python Code To Draw A House
import turtle
t = turtle.Turtle()
# Create a new screen for the turtle and set the background color
screen = turtle.Screen()
screen.bgcolor("#f9fafc")
# Set the turtle color, shape, and speed
t.color("black")
t.shape("turtle")
t.speed(2)
# Draw the base of the house
t.fillcolor('yellow')
t.begin_fill()
t.right(90) # turn the turtle right by 90 degrees
t.forward(250) # move the turtle forward by 250 units
t.left(90) # turn the turtle left by 90 degrees
t.forward(400) # move the turtle forward by 400 units
t.left(90) # turn the turtle left by 90 degrees
t.forward(250) # move the turtle forward by 250 units
t.left(90) # turn the turtle left by 90 degrees
t.forward(400) # move the turtle forward by 400 units
t.right(90) # turn the turtle right by 90 degrees
t.end_fill() # end the fill color
# Draw the top of the house
t.fillcolor('red')
t.begin_fill()
t.right(45) # turn the turtle right by 45 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(200) # move the turtle forward by 200 units
t.left(180) # turn the turtle left by 180 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(135) # turn the turtle right by 135 degrees
t.forward(259) # move the turtle forward by 259 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(142) # move the turtle forward by 142 units
t.end_fill() # end the fill color
# Draw the door and windows
t.right(90) # turn the turtle right by 90 degrees
t.forward(400) # move the turtle forward by 400 units
t.left(90) # turn the turtle left by 90 degrees
t.forward(50) # move the turtle forward by 50 units
t.left(90) # turn the turtle left by 90 degrees
t.forward(150) # move the turtle forward by 150 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(180) # turn the turtle left by 180 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(150) # move the turtle forward by 150 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(150) # move the turtle forward by 150 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(100) # move the turtle forward by 100 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(150) # move the turtle forward by 150 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(100) # move the turtle forward by 100 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(75) # move the turtle forward by 75 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(180) # turn the turtle left by 180 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(75) # move the turtle forward by 75 units
t.left(90) # turn the turtle left by 90 degrees
t.forward(15) # move the turtle forward by 15 units
t.left(90) # turn the turtle left by 90 degrees
t.forward(200) # move the turtle forward by 200 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(15) # move the turtle forward by 15 units
t.right(90) # turn the turtle right by 90 degrees
t.forward(75) # move the turtle forward by 75 units
turtle.done()
Above is the python program to draw a house Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or else you can run on this online python compiler.
As we have used the turtle library for this program so you might need to install it, mostly it is preinstalled but incase if you get any errors like turtle module not found then use below command to install.
pip install turtle
So now you have everything setup and you are ready to run the program, so to run this program open a terminal at your program folder location and paste the below command.
python filename.py
The above command will run the program and it will open a new window and it will start drawing a house and below is the finished drawing of the house.
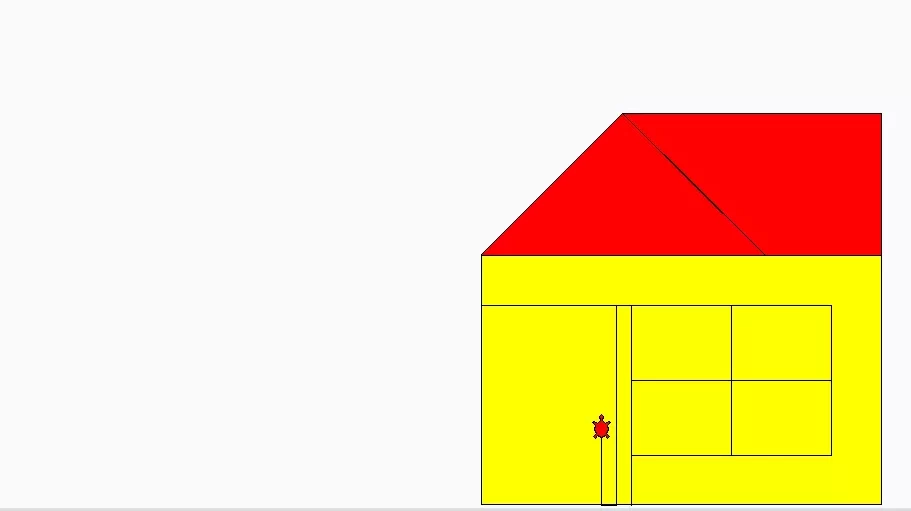
As you can see it successfully drew a house, so this was the tutorial I hope you found this tutorial helpful and useful. Do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw Avengers Logo Using Python Turtle.
I hope you found what you were looking for from this tutorial, and if you want more python resources and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂