Draw Panda In Python Using Turtle Module
Last updated July 3, 2023 by Jarvis Silva
Want to know how to draw panda in python then you are at the right place, In this tutorial we will use our python programming skills to draw panda.
To draw a panda we will use the turtle module in python,It is a GUI library which can be used to draw anything from animals, characters, cartoons, shapes and other objects.
Python Code To Draw Panda
import turtle
t = turtle.Turtle()
turtle.Screen().bgcolor("yellow")
def circles(color, radius):
# Set the fill
t.fillcolor(color)
# Start filling the color
t.begin_fill()
# Draw a circle
t.circle(radius)
# Ending the filling of the color
t.end_fill()
# Draw first ear
t.up()
t.setpos(-35, 95)
t.down
circles('black', 15)
# Draw second ear
t.up()
t.setpos(35, 95)
t.down()
circles('black', 15)
#....... Draw face ......#
t.up()
t.setpos(0, 35)
t.down()
circles('white', 40)
#....... Draw eyes black .....#
# Draw first eye
t.up()
t.setpos(-18, 75)
t.down
circles('black', 8)
# Draw second eye
t.up()
t.setpos(18, 75)
t.down()
circles('black', 8)
#........ Draw eyes white ......#
# Draw first eye
t.up()
t.setpos(-18, 77)
t.down()
circles('white', 4)
# Draw second eye
t.up()
t.setpos(18, 77)
t.down()
circles('white', 4)
#......... Draw nose......#
t.up()
t.setpos(0, 55)
t.down
circles('black', 5)
#...... Draw mouth ....#
t.up()
t.setpos(0, 55)
t.down()
t.right(90)
t.circle(5, 180)
t.up()
t.setpos(0, 55)
t.down()
t.left(360)
t.circle(5, -180)
t.hideturtle()
Above is the python program to draw a panda as you can see it is quite long code so let’s see how exactly does this code work:
- Importing the
turtle
module and creating a turtle object namedt
. - Setting the background color of the turtle screen to yellow.
- Defining a function called
circles
that takes a color and radius as parameters. This function will be used to draw filled circles. - Inside the
circles
function:- Setting the fill color to the provided color.
- Starting the filling of the color.
- Drawing a circle with the given radius.
- Ending the filling of the color.
- Drawing the first ear:
- Lifting the turtle pen up.
- Moving the turtle to the position (-35, 95).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with black color and radius of 15.
- Drawing the second ear:
- Lifting the turtle pen up.
- Moving the turtle to the position (35, 95).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with black color and radius of 15.
- Drawing the face:
- Lifting the turtle pen up.
- Moving the turtle to the position (0, 35).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with white color and radius of 40.
- Drawing the black eyes:
- Drawing the first eye:
- Lifting the turtle pen up.
- Moving the turtle to the position (-18, 75).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with black color and radius of 8.
- Drawing the second eye:
- Lifting the turtle pen up.
- Moving the turtle to the position (18, 75).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with black color and radius of 8.
- Drawing the first eye:
- Drawing the white eye highlights:
- Drawing the first eye highlight:
- Lifting the turtle pen up.
- Moving the turtle to the position (-18, 77).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with white color and radius of 4.
- Drawing the second eye highlight:
- Lifting the turtle pen up.
- Moving the turtle to the position (18, 77).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with white color and radius of 4.
- Drawing the first eye highlight:
- Drawing the nose:
- Lifting the turtle pen up.
- Moving the turtle to the position (0, 55).
- Putting the pen down.
- Calling the
circles
function to draw a filled circle with black color and radius of 5.
- Drawing the mouth:
- Lifting the turtle pen up.
- Moving the turtle to the position (0, 55).
- Putting the pen down.
- Turning the turtle right by 90 degrees.
- Drawing a semicircle with a radius of 5, representing the upper part of the mouth.
- Lifting the turtle pen up.
- Moving the turtle to the position (0, 55).
- Putting the pen down.
- Turning the turtle left by 360 degrees.
- Drawing a semicircle with a radius of 5, representing the lower part of the mouth.
- Finally, the program ends with
turtle.done()
, which keeps the turtle graphics window open.
Now you can run this program using an online python compiler or on your device for that you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer.
As we have used turtle module to create this program you might need to install it if you get any errors saying turtle module not found use below command to install.
pip install turtle
So finally when you run this program it will open a new window and start drawing a cute panda, below is the finished drawing of a panda.
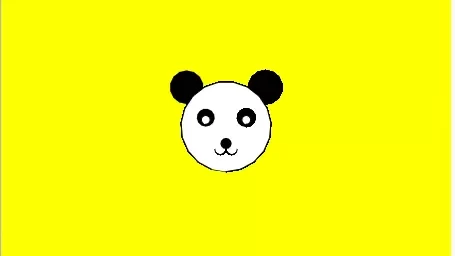
As you can see, we successfully drew a cute panda face using python turtle, I hope you found this tutorial helpful and useful, Do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂