Draw Smiley Face In Python With Turtle
Last updated July 3, 2023 by Jarvis Silva
In this tutorial I will show you how to draw a smiley face in python turtle, We will use the turtle module in python to draw smiley face, turtle is a GUI library which can be used to draw anything from characters, cartoons, shapes and other objects.
Python Code To Draw Smiley Face
import turtle as t
t.pensize(10)
t.fillcolor('yellow')
t.hideturtle()
t.bgcolor("#eeeeee")
radius = 180
t.penup()
t.setposition(0, -radius)
t.setheading(0)
t.pendown()
t.begin_fill()
t.circle(radius)
t.end_fill()
mouth_radius = radius * 0.6
mouth_angle = 70
t.penup()
t.setposition(0, -mouth_radius)
t.setheading(0)
t.pendown()
t.circle(mouth_radius, mouth_angle)
t.penup()
t.setposition(0, -mouth_radius)
t.setheading(0)
t.pendown()
t.circle(mouth_radius, -mouth_angle)
eye_x = 50
eye_y = 50
eye_size = 60
t.penup()
t.setposition(eye_x, eye_y)
t.pendown()
t.dot(eye_size)
t.penup()
t.setposition(-eye_x, eye_y)
t.pendown()
t.dot(eye_size)
t.done()
Above is the code for drawing smiley face emoji, the complete code is made using turtle functions so let’s see how they work:
- The code uses the Turtle graphics library to create a drawing of a smiley face.
- The turtle is configured with a pen size of 10 and a yellow fill color.
- The turtle’s appearance is hidden, and the background color is set to light gray.
- A large circle with a radius of 180 is drawn, representing the face of the smiley.
- The mouth of the smiley is drawn using an arc with a smaller radius and a specific angle.
- Two eyes are drawn as dots on the face, positioned symmetrically.
- The turtle’s pen is lifted up and moved to different positions to draw the various elements of the smiley.
- The fill color is used to color the smiley’s face and complete the drawing.
Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or use this online python compiler.
After you run the program and it will open a new window and it will start drawing a smiley face and below is the finished drawing of a smiley face emoji.
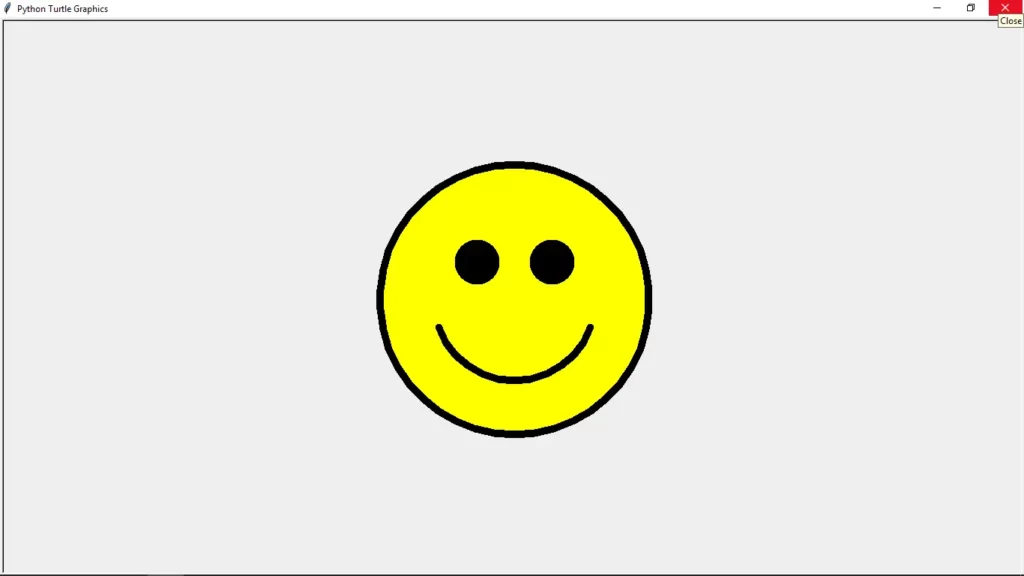
As you can see, we successfully drew a smiley face using python turtle, I hope you found this tutorial helpful and useful, Do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
- Draw Panda In Python Turtle With Code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂