Draw Netflix Logo Using Python Turtle Library
Last updated July 3, 2023 by Jarvis Silva
Want to know how to draw a Netflix logo using python then you are at the right place, follow this tutorial till the end to have netflix logo drawing program.
Netflix is one of the most popular ott platform to watch web series and movies, so I have decided to draw its logo using my python skills.
We will use the turtle module to draw the Netflix logo,Turtle is a GUI library with help of this library you can draw anything in python.
Python Code To Draw Netflix Logo
# import the turtle module and time module for delay
import turtle
from time import sleep
# create a turtle object
t = turtle.Turtle()
# set the speed of the turtle
t.speed(4)
# set the background color of the screen
turtle.bgcolor("white")
# set the color of the turtle
t.color("white")
# set the title of the window
turtle.title('Netflix Logo')
# move the turtle to the starting position
t.up()
t.goto(-80, 50)
t.down()
# draw the outer box of the logo
t.fillcolor("black")
t.begin_fill()
t.forward(200)
t.setheading(270)
s = 360
for i in range(9):
s = s - 10
t.setheading(s)
t.forward(10)
t.forward(180)
s = 270
for i in range(9):
s = s - 10
t.setheading(s)
t.forward(10)
t.forward(200)
s = 180
for i in range(9):
s = s - 10
t.setheading(s)
t.forward(10)
t.forward(180)
s = 90
for i in range(9):
s = s - 10
t.setheading(s)
t.forward(10)
t.forward(30)
t.end_fill()
# draw the red stripe in the logo
t.up()
t.color("black")
t.setheading(270)
t.forward(240)
t.setheading(0)
t.down()
t.color("red")
t.fillcolor("#E50914")
t.begin_fill()
t.forward(30)
t.setheading(90)
t.forward(180)
t.setheading(180)
t.forward(30)
t.setheading(270)
t.forward(180)
t.end_fill()
t.setheading(0)
t.up()
t.forward(75)
t.down()
t.color("red")
t.fillcolor("#E50914")
t.begin_fill()
t.forward(30)
t.setheading(90)
t.forward(180)
t.setheading(180)
t.forward(30)
t.setheading(270)
t.forward(180)
t.end_fill()
# draw the "N" in the logo
t.color("red")
t.fillcolor("red")
t.begin_fill()
t.setheading(113)
t.forward(195)
t.setheading(0)
t.forward(31)
t.setheading(293)
t.forward(196)
t.end_fill()
# hide the turtle and wait for 10 seconds before closing the window
t.hideturtle()
sleep(10)
turtle.done()
There is the code to draw the Netflix logo. Python installation is required to run the program If you don’t have then follow this guide: Install and setup python on your computer or else you can use this online compiler to run it.
Now you have the code, but there is one last thing you need to do as we have used turtle library to create this program so you might need to install it, mostly it is pre-intalled but if you get any errors like turtle module not found then use below command to install.
pip install turtle
So now you have everything setup and you are ready to run the program, to run this program open a command prompt at your program folder location and paste the below command.
python filename.py
The above command will open a new window and start drawing the Netflix logo. The finished drawing will be displayed in the window below is the output you should get.
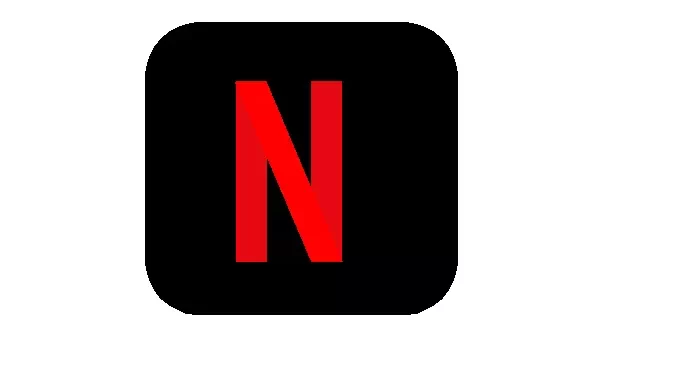
As you can see, we successfully drew the Netflix using python turtle. I hope you were able to follow along and execute the program successfully, do share this tutorial with your friends who might be interested in this program.
Want more amazing turtle tutorials like this check out this: Awesome Python Turtle Codes.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Create a fruit ninja game in python.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
- Draw car in python turtle with code.
- Draw a Cat In Python Turtle With Code.
- Draw Panda In Python Turtle With Code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂