Draw Avengers Logo Using Python
Last updated July 3, 2023 by Jarvis Silva
If you’re looking to draw the Avengers logo using Python, you’re in the right place, follow the steps shown in this tutorial to draw your own Avengers logo.
Avengers is one of the most popular superhero franchise in the world. I am a fan of avengers movies, so today as a fan I decided to create this tutorial on drawing avengers logo in python.
Python Code To Draw Avengers Logo
# Import the turtle module
from turtle import *
# Create a turtle object named 'drawer'
drawer = Turtle()
# Create a screen object named 's'
s = Screen()
# Set the background color of the screen to black
s.bgcolor("black")
# Lift the pen and move it to the starting position for the outer circle
drawer.penup()
drawer.setposition(-20,-350)
# Lower the pen and begin filling the circle with red color
drawer.pendown()
drawer.begin_fill()
drawer.color("red")
drawer.circle(300)
drawer.end_fill()
# Lift the pen and move it to the starting position for the inner circle
drawer.penup()
drawer.setposition(-20,-260)
# Lower the pen and begin filling the circle with black color
drawer.pendown()
drawer.begin_fill()
drawer.color("black")
drawer.circle(230)
drawer.end_fill()
# Lift the pen and move it to the starting position for the 'A' shape
drawer.penup()
drawer.setposition(0,-100)
# Lower the pen and begin filling the 'A' shape with red color
drawer.pendown()
drawer.begin_fill()
drawer.color("red")
# Set the pen size and color
drawer.pensize(2)
drawer.pencolor("grey")
# Draw the 'A' shape
drawer.backward(100)
drawer.left(60)
drawer.backward(200)
drawer.right(60)
drawer.backward(85)
drawer.right(115)
drawer.backward(600)
drawer.right(65)
drawer.backward(130)
drawer.right(90)
drawer.backward(440)
drawer.right(90)
drawer.backward(100)
drawer.right(90)
drawer.backward(65)
# End the fill for the 'A' shape
drawer.end_fill()
# Lift the pen and move it to the starting position for the 'V' shape
drawer.penup()
drawer.setposition(0,-50)
# Lower the pen and begin filling the 'V' shape with black color
drawer.pendown()
drawer.pensize(2)
drawer.begin_fill()
drawer.color("black")
drawer.pencolor("grey")
# Draw the 'V' shape
drawer.right(90)
drawer.forward(90)
drawer.right(120)
drawer.forward(170)
drawer.right(150)
drawer.forward(150)
# End the fill for the 'V' shape
drawer.end_fill()
# Keep the screen open after the logo has been drawn
done()
Above is the python program to draw the avengers logo. Now to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer.
I have used the turtle module in python to draw the avengers logo so you need to install it if it is not installed you can do so by using below command.
pip install turtle
So now you have everything setup and you are ready to run the program, so to run this program open a command prompt at your program folder location and paste the below command.
python filename.py
The above command will run the program and it will open a new window and it will start drawing the logo and below is the finished drawing of the avengers logo.
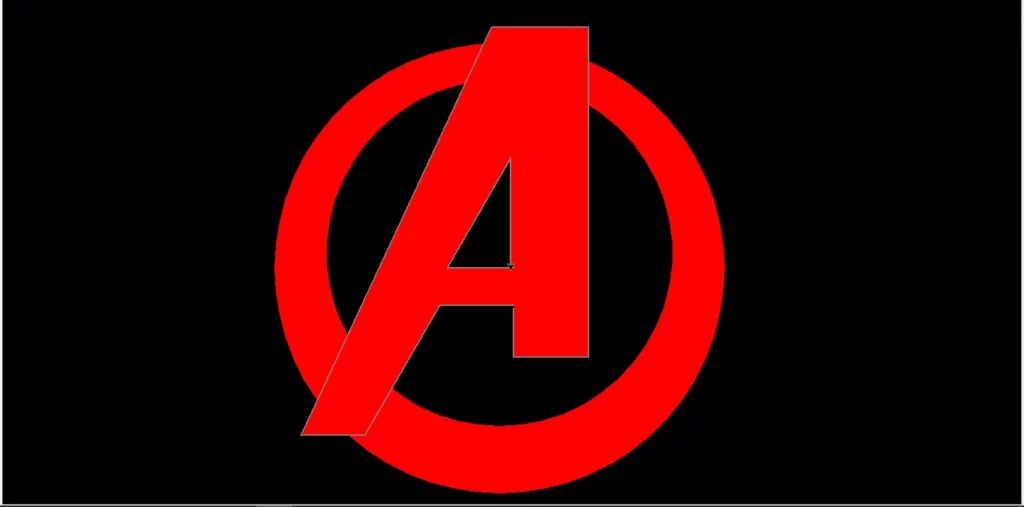
As you can see, we successfully drew the avengers logo it looks exactly like it. Don’t want to create all the files and folders then you can run this program now using this online python compiler it is fast and easy.
This was for this tutorial I hope you found this tutorial helpful and useful. Do share this tutorial with your friends who might be interested in this program especially marvel fans.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂