Draw Christmas Tree In Python Turtle
Last updated July 3, 2023 by Jarvis Silva
In this tutorial we wil draw christmas tree in python, you will be able use this program to wish merry christmas to your family & friends, this program will draw a christmas tree and it will have merry christmas written at the bottom.
To create this program we will use turtle which is a python library for GUI, You can draw stuff with this library.
Python Code To Draw Christmas Tree
from turtle import *
speed(0)
# Blue Background
penup()
goto(0, -250)
pendown()
color("lightskyblue")
begin_fill()
circle(250)
end_fill()
# Tree Trunk
penup()
goto(-15, -50)
pendown()
color("brown")
begin_fill()
for i in range(2):
forward(30)
right(90)
forward(40)
right(90)
end_fill()
# Set the start position and the inital tree width
y = -50
width = 240
height = 25
# Green section of tree (add in the greater than symbol next to the 20 - YouTube doesn't allow me to put in pointy brackets).
while width > 20:
width = width - 30 # Make the tree get smaller as it goes up in height
x = 0 - width / 2 # Set the starting x-value of each level of the tree
color("green")
penup()
goto(x, y)
pendown()
begin_fill()
for i in range(2):
forward(width)
left(90)
forward(height)
left(90)
end_fill()
y = y + height # Keep drawing the levels of the tree higher than the previous
# Star
penup()
goto(-15, 150)
pendown()
color("yellow")
begin_fill()
for i in range(5):
forward(30)
right(144)
end_fill()
# Message
penup()
goto(-130, -150)
color("red")
write("MERRY CHRISTMAS", font=("Arial", 20, "bold"))
hideturtle()
Above is the python code for drawing christmas tree, if you take a look at the code it entirely created using turtle functions so let’s see how this code works:
- The code starts by importing the
Turtle
class from theturtle
module. - The
speed(0)
function call sets the drawing speed to the fastest. - The code begins drawing a blue background by moving the pen to the bottom center of the screen and drawing a filled circle with a radius of 250 using the “lightskyblue” color.
- Next, the tree trunk is drawn by moving the pen to the base of the tree and drawing a filled rectangle with a width of 30 and a height of 40 using the “brown” color.
- The variables
y
,width
, andheight
are initialized to control the dimensions and positioning of the tree levels. - The green section of the tree is drawn using a while loop that continues as long as the
width
is greater than 20. In each iteration, thewidth
is reduced by 30 to make the tree narrower as it goes up. Thex
variable is set to position each level of the tree horizontally. The pen is moved to the starting position, and a filled rectangle is drawn with a width ofwidth
and a height ofheight
. They
value is incremented to position the next level higher than the previous one. - The star at the top of the tree is drawn by moving the pen to a specific position and drawing a filled star shape with a size of 30 units for each of the five points using the “yellow” color.
- Finally, the “MERRY CHRISTMAS” message is written on the screen using the pen’s
write()
function, positioned at (-130, -150) with a red color and a font of Arial, size 20, and bold style. - The
hideturtle()
function call hides the turtle cursor.
You can run this program on your computer or you can use an online compiler, as soon as you run it will open a new window and it will start to draw a christmas tree, after finishing below is the output you should get.
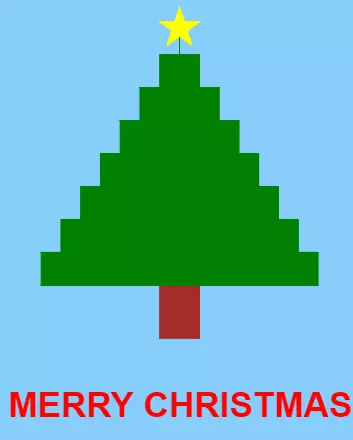
As you can see it draws a christmas tree and has merry christmas beneath it, do wish your friends a merry christmas by using this python program they will surely get surprised by it, specially if your friend is a programmer.
Here are some more python guides that might interest you:
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
I hope you found what you were looking for from this tutorial. If you want more python tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, Merry Christmas 🙂