Draw Flower In Python Using Turtle
Last updated July 3, 2023 by Jarvis Silva
Looking for a tutorial on how to draw flower using python then you are at the right place, in this tutorial we will create a python program to draw a petal and a rose flower if you want to know how to draw them follow along.
To draw these flowers in python we will use the turtle module, it is graphics library which allows us to draw character, animations etc.
Python Code To Draw Petal Flower
import turtle
# Set the title of the Turtle window
turtle.title('Sixteen Petals Flower')
# Set the world coordinates for the Turtle canvas
turtle.setworldcoordinates(-2000,-2000,2000,2000)
# Define a function called "draw_flower" that takes four arguments: x, y, tilt, and radius
def draw_flower(x, y, tilt, radius):
# Move the turtle's pen up (so it doesn't draw a line when moving)
turtle.up()
# Move the turtle to the given x,y coordinates
turtle.goto(x, y)
# Move the turtle's pen down (so it can draw a line when moving)
turtle.down()
# Set the turtle's heading to the given tilt angle minus 45 degrees
turtle.seth(tilt-45)
# Draw a partial circle with the given radius, starting at the turtle's current position and heading
# and spanning 90 degrees (i.e. a quarter of a circle)
turtle.circle(radius, 90)
# Turn the turtle left 90 degrees
turtle.left(90)
# Draw another partial circle with the given radius, starting at the turtle's current position and heading
# and spanning another 90 degrees (i.e. the second quarter of a circle, completing a half-circle in total)
turtle.circle(radius, 90)
# Loop through a range of angles from 0 to 360 degrees in steps of 30 degrees
for tilt in range(0, 360, 30):
# Call the "draw_flower" function with x=0, y=0, the current tilt angle, and a radius of 1000
draw_flower(0, 0, tilt, 1000)
Above is the code for drawing a petal flower, I have added comments to each line of code so that you know what each line does.
As we have used the turtle library you might need to install it before you can run the program, mostily turtle is preinstalled with python but if you get any errors like turtle module not found then you use the below command to install
pip install turtle
Now you can run the above program on your computer or use this online python compiler and below is the output you should get after running this program.
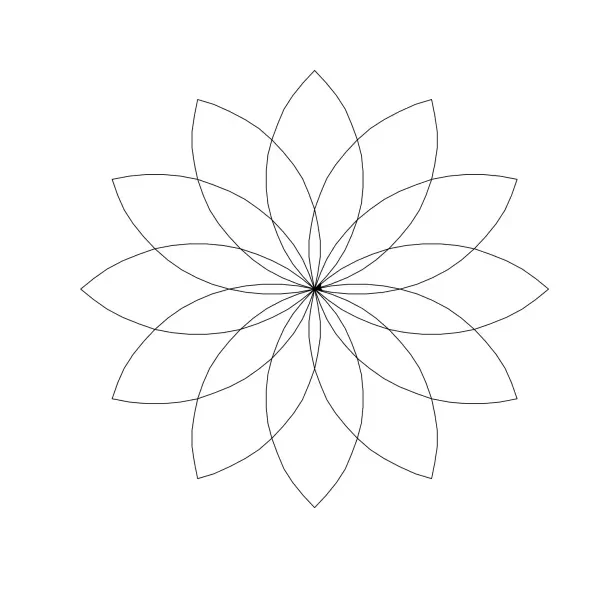
As you can see we have successfully drawn a petal flower now let’s see how to draw a rose in python.
Python Code To Draw a Rose Flower
from turtle import *
import turtle as t
t = t.Turtle()
t.penup ()
t.left (90)
t.fd (200)
t.pendown ()
t.right (90)
t.fillcolor ("red")
t.begin_fill ()
t.circle (10,180)
t.circle (25,110)
t.left (50)
t.circle (60,45)
t.circle (20,170)
t.right (24)
t.fd (30)
t.left (10)
t.circle (30,110)
t.fd (20)
t.left (40)
t.circle (90,70)
t.circle (30,150)
t.right (30)
t.fd (15)
t.circle (80,90)
t.left (15)
t.fd (45)
t.right (165)
t.fd (20)
t.left (155)
t.circle (150,80)
t.left (50)
t.circle (150,90)
t.end_fill ()
t.left (150)
t.circle (-90,70)
t.left (20)
t.circle (75,105)
t.setheading (60)
t.circle (80,98)
t.circle (-90,40)
t.left (180)
t.circle (90,40)
t.circle (-80,98)
t.setheading (-83)
t.fd (30)
t.left (90)
t.fd (25)
t.left (45)
t.fillcolor ("dark green")
t.begin_fill ()
t.circle (-80,90)
t.right (90)
t.circle (-80,90)
t.end_fill ()
t.right (135)
t.fd (60)
t.left (180)
t.fd (85)
t.left (90)
t.fd (80)
t.right (90)
t.right (45)
t.fillcolor ("dark green")
t.begin_fill ()
t.circle (80,90)
t.left (90)
t.circle (80,90)
t.end_fill ()
t.left (135)
t.fd (60)
t.left (180)
t.fd (60)
t.right (90)
t.circle (200,60)
t.done()
Above is the code for drawing a rose in python, follow the steps which I told previously in how to draw a petal flower to run this program and below is the output you should get
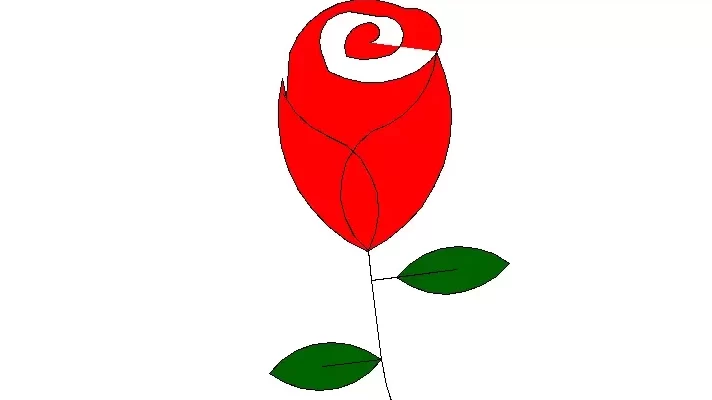
As you can see it draws a beautiful red rose, if you know how to use the turtle module then you can make it more beautiful so now you know to draw two types of flowers in python.
Here are more python drawing tutorials for you:
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Captain America shield in python.
- Draw American flag in python turtle.
I hope you found what you were looking for from this python tutorial, do share this tutorial with your friends who might be interested and If you want more python tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂