Draw Car In Python Turtle
Last updated July 3, 2023 by Jarvis Silva
Today in this tutorial we will see how to draw a car using python programming to create this program we will use the turtle module in python, It is a GUI python library which can be used to draw anything from characters, cartoons, shapes and other objects.
Python Code To Draw A Car
Above is the python program to draw a car, as you can see from the code it is all done with the turtle module so let’s understand how the code works:
- Importing the
turtle
module to use its functions and classes. - Creating a turtle object named
myCar
. - Drawing the rectangular upper body of the car:
- Setting the color to black and fill color to yellow.
- Moving the turtle to the starting position (0, 0).
- Beginning the fill.
- Moving the turtle forward by 370 units (length of the body).
- Turning left by 90 degrees.
- Moving the turtle forward by 50 units (height of the body).
- Turning left by 90 degrees.
- Moving the turtle forward by 370 units.
- Turning left by 90 degrees.
- Moving the turtle forward by 50 units.
- Ending the fill.
- Drawing the window and roof of the car:
- Moving the turtle to the position (100, 50).
- Setting the heading to 45 degrees.
- Moving the turtle forward by 70 units.
- Setting the heading to 0 degrees.
- Moving the turtle forward by 100 units.
- Setting the heading to -45 degrees.
- Moving the turtle forward by 70 units.
- Setting the heading to 90 degrees.
- Moving the turtle to the position (200, 50).
- Moving the turtle forward by 49.50 units (width of the roof).
- Drawing the two tires:
- Moving the turtle to the position (100, -10).
- Setting the color and fill color to black.
- Beginning the fill.
- Drawing a circle with a radius of 20 units.
- Ending the fill.
- Moving the turtle to the position (300, -10).
- Setting the color and fill color to black.
- Beginning the fill.
- Drawing a circle with a radius of 20 units.
- Ending the fill.
- Hiding the turtle to only display the car.
- Ending the program with
turtle.done()
, which keeps the turtle graphics window open.
Now you know how the code works let’s run it to run this program you need to have python installed on your computer, If you don’t have then follow this guide: Install and setup python on your computer or else you can use this online python compiler
Now you have the code, but there is one last thing you might need to do as we have used the turtle library for this program so you might need to install it if you get any errors like turtle module not found use below command to install.
After running this program it will open a new window and it will start drawing the car and below is the finished drawing of the car.
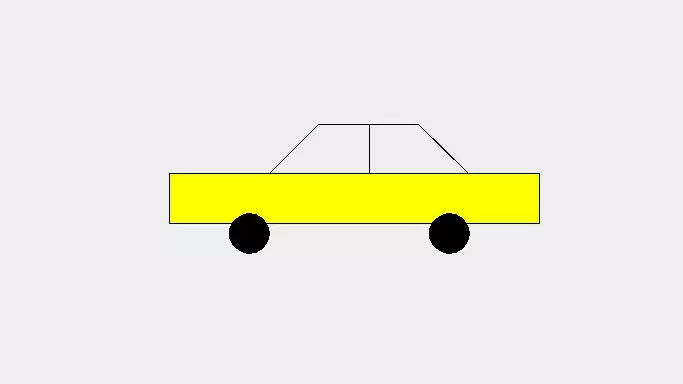
As you can see, we successfully drew the car using python turtle. I hope you were able to run this program successfully, do share this tutorial with your friends who might be interested in this program.
Here are some more python drawing tutorials for you:
- Draw Pikachu using python with code.
- Draw doraemon using python turtle.
- Draw shinchan using python turtle.
- Draw I love you using python turtle.
- Draw Batman logo using python turtle.
- Draw Google Logo using python turtle.
- Make a python calculator using turtle.
- Draw christmas tree using python.
- Draw spiderman in python programming.
- Draw Python Logo Using Python.
- Draw Iron Man using python turtle with code.
- Draw A Heart Using python turtle with code.
I hope you found what you were looking for from this tutorial, and if you want more python guides and tutorials like this, do join our Telegram channel for future updates.
Thanks for reading, have a nice day 🙂