Python Program To Wish Happy Birthday With Code
Last updated June 6, 2023 by Jarvis Silva
Your friend’s birthday is coming and you want to wish him differently and you both are programmers so you want to do it in a programmers way then in this tutorial we will create a python program to wish happy birthday.
To make this program we will use some libraries first is turtle which is used to draw graphics in python and also we will use pygame for adding some happy birthday music to the program.
Python Code To Wish Happy Birthday
import turtle
import random
import time
from pygame import mixer
# Adding music is optional as per your choice.
mixer.pre_init(frequency=48000, size=-16, channels=2, buffer=512)
mixer.init()
mixer.music.load("happy-birthday-song.mp3") #add your music file name or path
# sets background
bg = turtle.Screen()
bg.bgcolor("black")
mixer.music.play()
# Bottom Line 1
turtle.penup()
turtle.goto(-170,-180)
turtle.color("white")
turtle.pendown()
turtle.forward(350)
# Mid Line 2
turtle.penup()
turtle.goto(-160,-150)
turtle.color("white")
turtle.pendown()
turtle.forward(300)
# First Line 3
turtle.penup()
turtle.goto(-150,-120)
turtle.color("white")
turtle.pendown()
turtle.forward(250)
bg.bgcolor("lightgreen")
# Cake
turtle.penup()
turtle.goto(-100,-100)
turtle.color("white")
turtle.begin_fill()
turtle.pendown()
turtle.forward(140)
turtle.left(90)
turtle.forward(95)
turtle.left(90)
turtle.forward(140)
turtle.left(90)
turtle.forward(95)
turtle.end_fill()
bg.bgcolor("lightblue")
# Candles
turtle.penup()
turtle.goto(-90,0)
turtle.color("red")
turtle.left(180)
turtle.pendown()
turtle.forward(20)
turtle.penup()
turtle.goto(-60,0)
turtle.color("blue")
turtle.pendown()
turtle.forward(20)
turtle.penup()
turtle.goto(-30,0)
turtle.color("yellow")
turtle.pendown()
turtle.forward(20)
turtle.penup()
turtle.goto(0,0)
turtle.color("green")
turtle.pendown()
turtle.forward(20)
turtle.penup()
turtle.goto(30,0)
turtle.color("purple")
turtle.pendown()
turtle.forward(20)
bg.bgcolor("orange")
# Decoration
colors = ["red", "orange", "yellow", "green", "blue", "purple", "black"]
turtle.penup()
turtle.goto(-40,-50)
turtle.pendown()
for each_color in colors:
angle = 360 / len(colors)
turtle.color(each_color)
turtle.circle(10)
turtle.right(angle)
turtle.forward(10)
bg.bgcolor("black")
# Happy Birthday message
turtle.penup()
turtle.goto(-150, 50)
turtle.color("pink")
turtle.pendown()
# ENTER YOUR NAME IN THE NAME PLACE
turtle.write(arg=f"Happy Birthday Name!", align="left", font=("jokerman", 24, "normal"))
time.sleep(5)
Copy the above code and paste it in your python file and get ready to run it, as I have said I used turtle and pygame, both of these are pre-installed with python but if you get any errors saying module not found then install it by using below commands.
# For installing turtle
pip install turtle
# For installing pygame
pip install pygame
Now just go into the code and find the below line of code and you need to enter the name of the person you want to wish.
# ENTER YOUR NAME IN THE NAME PLACE
turtle.write(arg=f"Happy Birthday Name!", align="left", font=("jokerman", 24, "normal"))
I have added a happy birthday song which will run while drawing the cake and saying happy birthday in this program, you can download the whole happy birthday python code and song below.
You can extract the above file and open it in a code editor of your choice, then you can run it if you don’t have python installed follow this guide: Install and setup python.
Final Happy Birthday Program Output
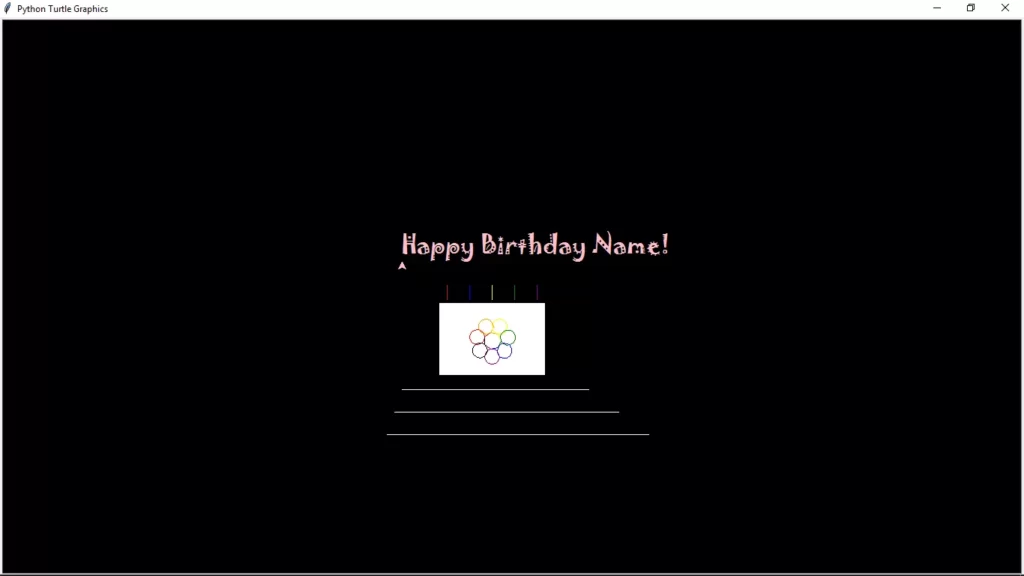
So this was an amazing tutorial on wishing happy birthday with python turtle, create this program and use it to wish friends and family they will surely love it.
Want more amazing python turtle programs, read these:
- Draw doraemon using python turtle with code.
- Draw shinchan using a python turtle with code.
- Draw I love you using a python turtle with code.
I hope you found this tutorial helpful and if you did then do share it with your programmers friends and family, do join our Telegram channel for future guides like this.
Thank you for reading, have a nice day 🙂